refactor the packages to remove internal dependency
This commit is contained in:
parent
ff7b4b38e2
commit
9900d6ea6d
16 changed files with 939 additions and 178 deletions
|
@ -1,3 +1,7 @@
|
|||
## 0.3.0
|
||||
|
||||
- Internal refactor
|
||||
|
||||
## 0.2.2
|
||||
|
||||
- Updated dependencies
|
||||
|
|
|
@ -1,12 +1,12 @@
|
|||
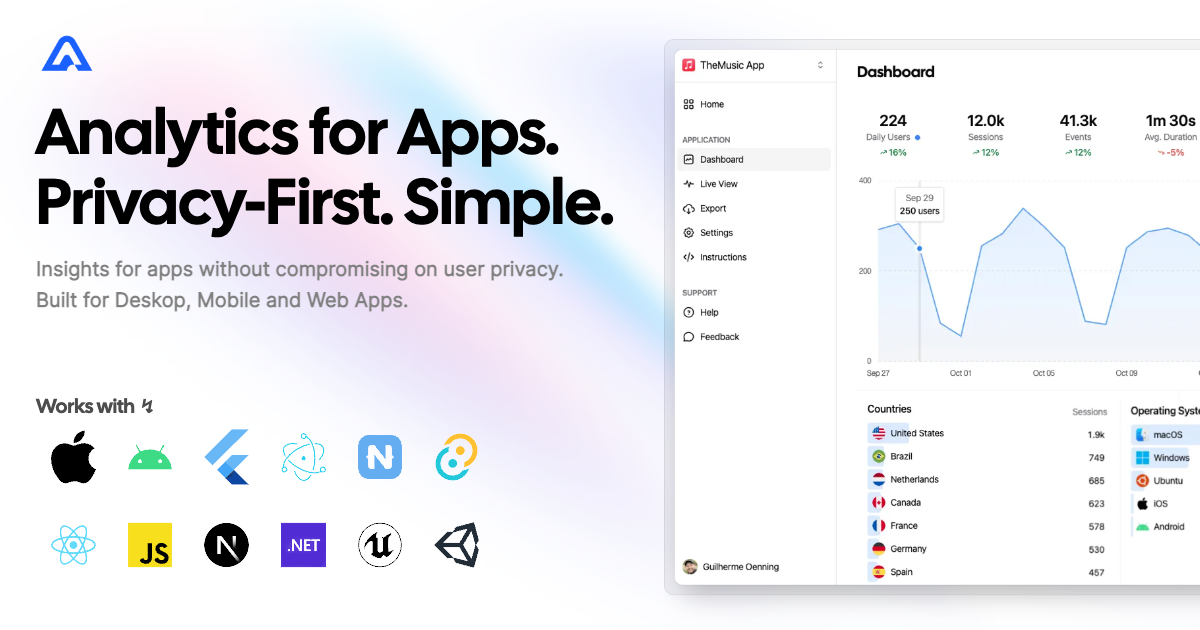
|
||||
|
||||
# React SDK for Aptabase
|
||||
# Aptabase SDK for React Apps
|
||||
|
||||
A tiny SDK (1 kB) to instrument your React apps with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
## Setup
|
||||
|
||||
1. Install the SDK using your preferred JavaScript package manager
|
||||
1. Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/react
|
||||
|
@ -105,6 +105,8 @@ ReactDOM.createRoot(root).render(
|
|||
|
||||
The `AptabaseProvider` also supports an optional second parameter, which is an object with the `appVersion` property.
|
||||
|
||||
It's up to you to decide how to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time. Alternatively you can also pass it in manually.
|
||||
|
||||
It's up to you to decide what to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time.
|
||||
|
||||
## Tracking Events with Aptabase
|
||||
|
@ -145,7 +147,7 @@ export function Counter() {
|
|||
|
||||
A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS, the app version, and other things.
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to await the `trackEvent` function, it'll run in the background.
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
{
|
||||
"name": "@aptabase/react",
|
||||
"version": "0.2.2",
|
||||
"version": "0.3.0",
|
||||
"type": "module",
|
||||
"description": "React SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"main": "./dist/index.cjs",
|
||||
|
@ -33,9 +33,6 @@
|
|||
"dist",
|
||||
"package.json"
|
||||
],
|
||||
"dependencies": {
|
||||
"@aptabase/web": "^0.3.0"
|
||||
},
|
||||
"peerDependencies": {
|
||||
"react": "^18.0.0"
|
||||
}
|
||||
|
|
1
packages/react/src/global.d.ts
vendored
1
packages/react/src/global.d.ts
vendored
|
@ -1 +0,0 @@
|
|||
declare var __APTABASE_SDK_VERSION__: string;
|
|
@ -1,18 +1,40 @@
|
|||
'use client';
|
||||
|
||||
import { init, trackEvent, type AptabaseOptions } from '@aptabase/web';
|
||||
import { createContext, useContext, useEffect } from 'react';
|
||||
import { inMemorySessionId, sendEvent, validateAppKey, type AptabaseOptions } from '../../shared';
|
||||
|
||||
globalThis.__APTABASE_SDK_VERSION__ = `aptabase-react@${process.env.PKG_VERSION}`;
|
||||
// Session expires after 1 hour of inactivity
|
||||
const SESSION_TIMEOUT = 1 * 60 * 60;
|
||||
const sdkVersion = `aptabase-react@${process.env.PKG_VERSION}`;
|
||||
|
||||
let _appKey = '';
|
||||
let _options: AptabaseOptions | undefined;
|
||||
|
||||
type ContextProps = {
|
||||
appKey?: string;
|
||||
options?: AptabaseOptions;
|
||||
};
|
||||
|
||||
export type AptabaseClient = {
|
||||
trackEvent: typeof trackEvent;
|
||||
};
|
||||
function init(appKey: string, options?: AptabaseOptions) {
|
||||
if (!validateAppKey(appKey)) return;
|
||||
|
||||
_appKey = appKey;
|
||||
_options = options;
|
||||
}
|
||||
|
||||
async function trackEvent(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
const sessionId = inMemorySessionId(SESSION_TIMEOUT);
|
||||
|
||||
await sendEvent({
|
||||
sessionId,
|
||||
appKey: _appKey,
|
||||
isDebug: _options?.isDebug,
|
||||
appVersion: _options?.appVersion,
|
||||
sdkVersion,
|
||||
eventName,
|
||||
props,
|
||||
});
|
||||
}
|
||||
|
||||
const AptabaseContext = createContext<ContextProps>({});
|
||||
|
||||
|
@ -22,6 +44,12 @@ type Props = {
|
|||
children: React.ReactNode;
|
||||
};
|
||||
|
||||
export { type AptabaseOptions };
|
||||
|
||||
export type AptabaseClient = {
|
||||
trackEvent: typeof trackEvent;
|
||||
};
|
||||
|
||||
export function AptabaseProvider({ appKey, options, children }: Props) {
|
||||
useEffect(() => {
|
||||
init(appKey, options);
|
||||
|
@ -33,17 +61,6 @@ export function AptabaseProvider({ appKey, options, children }: Props) {
|
|||
export function useAptabase(): AptabaseClient {
|
||||
const ctx = useContext(AptabaseContext);
|
||||
|
||||
if (typeof window === 'undefined') {
|
||||
return {
|
||||
trackEvent: (eventName: string) => {
|
||||
console.warn(
|
||||
`Aptabase: trackEvent can only be called from client components. Event '${eventName}' will be ignored.`,
|
||||
);
|
||||
return Promise.resolve();
|
||||
},
|
||||
};
|
||||
}
|
||||
|
||||
if (!ctx.appKey) {
|
||||
return {
|
||||
trackEvent: () => {
|
||||
|
@ -55,5 +72,7 @@ export function useAptabase(): AptabaseClient {
|
|||
};
|
||||
}
|
||||
|
||||
return { trackEvent };
|
||||
return {
|
||||
trackEvent,
|
||||
};
|
||||
}
|
||||
|
|
Loading…
Add table
Add a link
Reference in a new issue