refactor the packages to remove internal dependency
This commit is contained in:
parent
ff7b4b38e2
commit
9900d6ea6d
16 changed files with 939 additions and 178 deletions
|
@ -1,3 +1,7 @@
|
|||
## 0.3.0
|
||||
|
||||
- Internal refactor
|
||||
|
||||
## 0.2.2
|
||||
|
||||
- Updated dependencies
|
||||
|
|
|
@ -1,12 +1,12 @@
|
|||
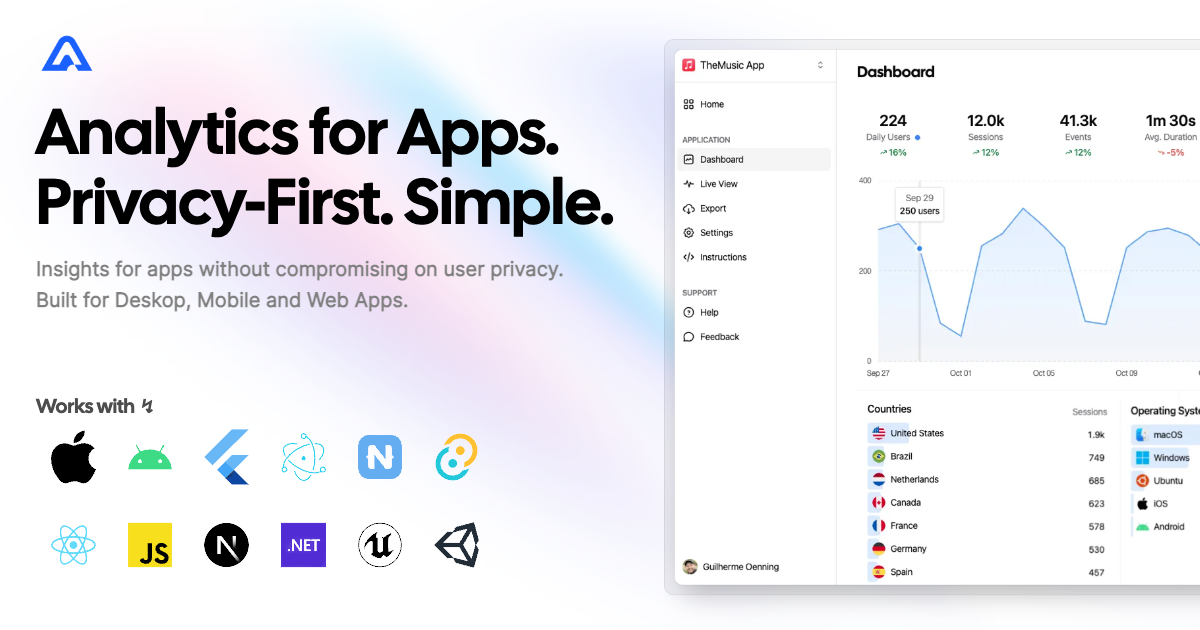
|
||||
|
||||
# React SDK for Aptabase
|
||||
# Aptabase SDK for React Apps
|
||||
|
||||
A tiny SDK (1 kB) to instrument your React apps with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
## Setup
|
||||
|
||||
1. Install the SDK using your preferred JavaScript package manager
|
||||
1. Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/react
|
||||
|
@ -105,6 +105,8 @@ ReactDOM.createRoot(root).render(
|
|||
|
||||
The `AptabaseProvider` also supports an optional second parameter, which is an object with the `appVersion` property.
|
||||
|
||||
It's up to you to decide how to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time. Alternatively you can also pass it in manually.
|
||||
|
||||
It's up to you to decide what to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time.
|
||||
|
||||
## Tracking Events with Aptabase
|
||||
|
@ -145,7 +147,7 @@ export function Counter() {
|
|||
|
||||
A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS, the app version, and other things.
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to await the `trackEvent` function, it'll run in the background.
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
{
|
||||
"name": "@aptabase/react",
|
||||
"version": "0.2.2",
|
||||
"version": "0.3.0",
|
||||
"type": "module",
|
||||
"description": "React SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"main": "./dist/index.cjs",
|
||||
|
@ -33,9 +33,6 @@
|
|||
"dist",
|
||||
"package.json"
|
||||
],
|
||||
"dependencies": {
|
||||
"@aptabase/web": "^0.3.0"
|
||||
},
|
||||
"peerDependencies": {
|
||||
"react": "^18.0.0"
|
||||
}
|
||||
|
|
1
packages/react/src/global.d.ts
vendored
1
packages/react/src/global.d.ts
vendored
|
@ -1 +0,0 @@
|
|||
declare var __APTABASE_SDK_VERSION__: string;
|
|
@ -1,18 +1,40 @@
|
|||
'use client';
|
||||
|
||||
import { init, trackEvent, type AptabaseOptions } from '@aptabase/web';
|
||||
import { createContext, useContext, useEffect } from 'react';
|
||||
import { inMemorySessionId, sendEvent, validateAppKey, type AptabaseOptions } from '../../shared';
|
||||
|
||||
globalThis.__APTABASE_SDK_VERSION__ = `aptabase-react@${process.env.PKG_VERSION}`;
|
||||
// Session expires after 1 hour of inactivity
|
||||
const SESSION_TIMEOUT = 1 * 60 * 60;
|
||||
const sdkVersion = `aptabase-react@${process.env.PKG_VERSION}`;
|
||||
|
||||
let _appKey = '';
|
||||
let _options: AptabaseOptions | undefined;
|
||||
|
||||
type ContextProps = {
|
||||
appKey?: string;
|
||||
options?: AptabaseOptions;
|
||||
};
|
||||
|
||||
export type AptabaseClient = {
|
||||
trackEvent: typeof trackEvent;
|
||||
};
|
||||
function init(appKey: string, options?: AptabaseOptions) {
|
||||
if (!validateAppKey(appKey)) return;
|
||||
|
||||
_appKey = appKey;
|
||||
_options = options;
|
||||
}
|
||||
|
||||
async function trackEvent(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
const sessionId = inMemorySessionId(SESSION_TIMEOUT);
|
||||
|
||||
await sendEvent({
|
||||
sessionId,
|
||||
appKey: _appKey,
|
||||
isDebug: _options?.isDebug,
|
||||
appVersion: _options?.appVersion,
|
||||
sdkVersion,
|
||||
eventName,
|
||||
props,
|
||||
});
|
||||
}
|
||||
|
||||
const AptabaseContext = createContext<ContextProps>({});
|
||||
|
||||
|
@ -22,6 +44,12 @@ type Props = {
|
|||
children: React.ReactNode;
|
||||
};
|
||||
|
||||
export { type AptabaseOptions };
|
||||
|
||||
export type AptabaseClient = {
|
||||
trackEvent: typeof trackEvent;
|
||||
};
|
||||
|
||||
export function AptabaseProvider({ appKey, options, children }: Props) {
|
||||
useEffect(() => {
|
||||
init(appKey, options);
|
||||
|
@ -33,17 +61,6 @@ export function AptabaseProvider({ appKey, options, children }: Props) {
|
|||
export function useAptabase(): AptabaseClient {
|
||||
const ctx = useContext(AptabaseContext);
|
||||
|
||||
if (typeof window === 'undefined') {
|
||||
return {
|
||||
trackEvent: (eventName: string) => {
|
||||
console.warn(
|
||||
`Aptabase: trackEvent can only be called from client components. Event '${eventName}' will be ignored.`,
|
||||
);
|
||||
return Promise.resolve();
|
||||
},
|
||||
};
|
||||
}
|
||||
|
||||
if (!ctx.appKey) {
|
||||
return {
|
||||
trackEvent: () => {
|
||||
|
@ -55,5 +72,7 @@ export function useAptabase(): AptabaseClient {
|
|||
};
|
||||
}
|
||||
|
||||
return { trackEvent };
|
||||
return {
|
||||
trackEvent,
|
||||
};
|
||||
}
|
||||
|
|
149
packages/shared.ts
Normal file
149
packages/shared.ts
Normal file
|
@ -0,0 +1,149 @@
|
|||
const defaultLocale = getBrowserLocale();
|
||||
const defaultIsDebug = getIsDebug();
|
||||
const isInBrowser = typeof window !== 'undefined' && typeof window.fetch !== 'undefined';
|
||||
|
||||
let _sessionId = newSessionId();
|
||||
let _lastTouched = new Date();
|
||||
|
||||
const apiUrl: Record<string, string> = {};
|
||||
|
||||
const _hosts: { [region: string]: string } = {
|
||||
US: 'https://us.aptabase.com',
|
||||
EU: 'https://eu.aptabase.com',
|
||||
DEV: 'http://localhost:3000',
|
||||
SH: '',
|
||||
};
|
||||
|
||||
export type AptabaseOptions = {
|
||||
host?: string;
|
||||
appVersion?: string;
|
||||
isDebug?: boolean;
|
||||
};
|
||||
|
||||
export function inMemorySessionId(timeout: number): string {
|
||||
let now = new Date();
|
||||
const diffInMs = now.getTime() - _lastTouched.getTime();
|
||||
const diffInSec = Math.floor(diffInMs / 1000);
|
||||
if (diffInSec > timeout) {
|
||||
_sessionId = newSessionId();
|
||||
}
|
||||
_lastTouched = now;
|
||||
|
||||
return _sessionId;
|
||||
}
|
||||
|
||||
export function newSessionId(): string {
|
||||
const epochInSeconds = Math.floor(Date.now() / 1000).toString();
|
||||
const random = Math.floor(Math.random() * 100000000)
|
||||
.toString()
|
||||
.padStart(8, '0');
|
||||
|
||||
return epochInSeconds + random;
|
||||
}
|
||||
|
||||
export function validateAppKey(appKey: string): boolean {
|
||||
const parts = appKey.split('-');
|
||||
if (parts.length !== 3 || _hosts[parts[1]] === undefined) {
|
||||
console.warn(`The Aptabase App Key "${appKey}" is invalid. Tracking will be disabled.`);
|
||||
return false;
|
||||
}
|
||||
return true;
|
||||
}
|
||||
|
||||
function getApiUrl(appKey: string, options?: AptabaseOptions): string | undefined {
|
||||
const url = apiUrl[appKey];
|
||||
if (url) url;
|
||||
|
||||
const region = appKey.split('-')[1];
|
||||
let host = _hosts[region];
|
||||
if (region === 'SH') {
|
||||
if (!options?.host) {
|
||||
console.warn(`Host parameter must be defined when using Self-Hosted App Key. Tracking will be disabled.`);
|
||||
return;
|
||||
}
|
||||
|
||||
host = options.host;
|
||||
}
|
||||
|
||||
apiUrl[appKey] = `${host}/api/v0/event`;
|
||||
return apiUrl[appKey];
|
||||
}
|
||||
|
||||
export async function sendEvent(opts: {
|
||||
appKey?: string;
|
||||
sessionId: string;
|
||||
locale?: string;
|
||||
isDebug?: boolean;
|
||||
appVersion?: string;
|
||||
sdkVersion: string;
|
||||
eventName: string;
|
||||
props?: Record<string, string | number | boolean>;
|
||||
}): Promise<void> {
|
||||
if (!isInBrowser) {
|
||||
console.warn(`Aptabase: trackEvent requires a browser environment. Event "${opts.eventName}" will be discarded.`);
|
||||
return;
|
||||
}
|
||||
|
||||
if (!opts.appKey) {
|
||||
console.warn(`Aptabase: init must be called before trackEvent. Event "${opts.eventName}" will be discarded.`);
|
||||
return;
|
||||
}
|
||||
|
||||
const apiUrl = getApiUrl(opts.appKey);
|
||||
if (!apiUrl) return;
|
||||
|
||||
try {
|
||||
const response = await fetch(apiUrl, {
|
||||
method: 'POST',
|
||||
headers: {
|
||||
'Content-Type': 'application/json',
|
||||
'App-Key': opts.appKey,
|
||||
},
|
||||
credentials: 'omit',
|
||||
body: JSON.stringify({
|
||||
timestamp: new Date().toISOString(),
|
||||
sessionId: opts.sessionId,
|
||||
eventName: opts.eventName,
|
||||
systemProps: {
|
||||
locale: opts.locale ?? defaultLocale,
|
||||
isDebug: opts.isDebug ?? defaultIsDebug,
|
||||
appVersion: opts.appVersion ?? '',
|
||||
sdkVersion: opts.sdkVersion,
|
||||
},
|
||||
props: opts.props,
|
||||
}),
|
||||
});
|
||||
|
||||
if (response.status >= 300) {
|
||||
const responseBody = await response.text();
|
||||
console.warn(`Failed to send event "${opts.eventName}": ${response.status} ${responseBody}`);
|
||||
}
|
||||
} catch (e) {
|
||||
console.warn(`Failed to send event "${opts.eventName}"`);
|
||||
console.warn(e);
|
||||
}
|
||||
}
|
||||
|
||||
function getBrowserLocale(): string | undefined {
|
||||
if (typeof navigator === 'undefined') {
|
||||
return undefined;
|
||||
}
|
||||
|
||||
if (navigator.languages.length > 0) {
|
||||
return navigator.languages[0];
|
||||
}
|
||||
|
||||
return navigator.language;
|
||||
}
|
||||
|
||||
function getIsDebug(): boolean {
|
||||
if (process.env.NODE_ENV === 'development') {
|
||||
return true;
|
||||
}
|
||||
|
||||
if (typeof location === 'undefined') {
|
||||
return false;
|
||||
}
|
||||
|
||||
return location.hostname === 'localhost';
|
||||
}
|
|
@ -1,3 +1,7 @@
|
|||
## 0.4.0
|
||||
|
||||
- Internal refactor
|
||||
|
||||
## 0.3.2
|
||||
|
||||
- better version of the session id generator
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
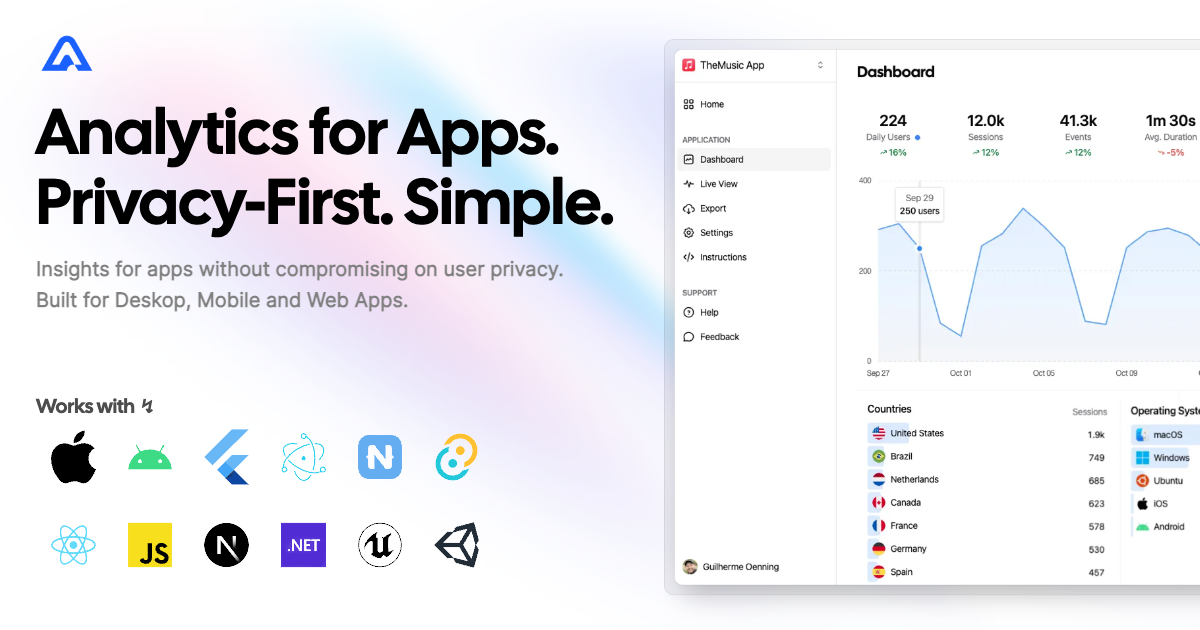
|
||||
|
||||
# JavaScript SDK for Aptabase
|
||||
# Aptabase SDK for Web Apps
|
||||
|
||||
A tiny SDK (1 kB) to instrument your web app with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
|
@ -12,7 +12,7 @@ Building a React app? Use the `@aptabase/react` package instead.
|
|||
|
||||
## Install
|
||||
|
||||
Install the SDK using your preferred JavaScript package manager
|
||||
Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/web
|
||||
|
@ -22,7 +22,7 @@ npm add @aptabase/web
|
|||
|
||||
First you need to get your `App Key` from Aptabase, you can find it in the `Instructions` menu on the left side menu.
|
||||
|
||||
Initialized the SDK using your `App Key`:
|
||||
Initialize the SDK using your `App Key`:
|
||||
|
||||
```js
|
||||
import { init } from '@aptabase/web';
|
||||
|
@ -32,7 +32,7 @@ init('<YOUR_APP_KEY>'); // 👈 this is where you enter your App Key
|
|||
|
||||
The init function also supports an optional second parameter, which is an object with the `appVersion` property.
|
||||
|
||||
It's up to you to decide what to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time.
|
||||
It's up to you to decide how to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time. Alternatively you can also pass it in manually.
|
||||
|
||||
Afterwards you can start tracking events with `trackEvent`:
|
||||
|
||||
|
@ -45,7 +45,7 @@ trackEvent('play_music', { name: 'Here comes the sun' }); // An event with a cus
|
|||
|
||||
A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS, the app version, and other things.
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to await the `trackEvent` function, it'll run in the background.
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
{
|
||||
"name": "@aptabase/web",
|
||||
"version": "0.3.2",
|
||||
"version": "0.4.0",
|
||||
"type": "module",
|
||||
"description": "JavaScript SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"main": "./dist/index.cjs",
|
||||
|
|
1
packages/web/src/global.d.ts
vendored
1
packages/web/src/global.d.ts
vendored
|
@ -1 +0,0 @@
|
|||
declare var __APTABASE_SDK_VERSION__: string;
|
|
@ -1,123 +1,31 @@
|
|||
import { newSessionId } from './session';
|
||||
|
||||
export type AptabaseOptions = {
|
||||
host?: string;
|
||||
appVersion?: string;
|
||||
isDebug?: boolean;
|
||||
};
|
||||
|
||||
const locale = getBrowserLocale();
|
||||
import { inMemorySessionId, sendEvent, validateAppKey, type AptabaseOptions } from '../../shared';
|
||||
|
||||
// Session expires after 1 hour of inactivity
|
||||
const SESSION_TIMEOUT = 1 * 60 * 60;
|
||||
let _sessionId = newSessionId();
|
||||
let _lastTouched = new Date();
|
||||
let _appKey = '';
|
||||
let _apiUrl = '';
|
||||
let _isDebug = false;
|
||||
let _appVersion = '';
|
||||
const sdkVersion = `aptabase-web@${process.env.PKG_VERSION}`;
|
||||
|
||||
const _hosts: { [region: string]: string } = {
|
||||
US: 'https://us.aptabase.com',
|
||||
EU: 'https://eu.aptabase.com',
|
||||
DEV: 'http://localhost:3000',
|
||||
SH: '',
|
||||
};
|
||||
let _appKey = '';
|
||||
let _options: AptabaseOptions | undefined;
|
||||
|
||||
export { type AptabaseOptions };
|
||||
|
||||
export function init(appKey: string, options?: AptabaseOptions) {
|
||||
const parts = appKey.split('-');
|
||||
if (parts.length !== 3 || _hosts[parts[1]] === undefined) {
|
||||
console.warn(`The Aptabase App Key "${appKey}" is invalid. Tracking will be disabled.`);
|
||||
return;
|
||||
}
|
||||
if (!validateAppKey(appKey)) return;
|
||||
|
||||
const baseUrl = getBaseUrl(parts[1], options);
|
||||
_apiUrl = `${baseUrl}/api/v0/event`;
|
||||
_appKey = appKey;
|
||||
_isDebug = options?.isDebug ?? getIsDebug();
|
||||
_appVersion = options?.appVersion ?? '';
|
||||
_options = options;
|
||||
}
|
||||
|
||||
export async function trackEvent(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
if (!_appKey) return;
|
||||
const sessionId = inMemorySessionId(SESSION_TIMEOUT);
|
||||
|
||||
if (typeof window === 'undefined' || !window.fetch) {
|
||||
console.warn(`Aptabase: trackEvent requires a browser environment. Event "${eventName}" will not be tracked.`);
|
||||
return;
|
||||
}
|
||||
|
||||
let now = new Date();
|
||||
const diffInMs = now.getTime() - _lastTouched.getTime();
|
||||
const diffInSec = Math.floor(diffInMs / 1000);
|
||||
if (diffInSec > SESSION_TIMEOUT) {
|
||||
_sessionId = newSessionId();
|
||||
}
|
||||
_lastTouched = now;
|
||||
|
||||
try {
|
||||
const response = await window.fetch(_apiUrl, {
|
||||
method: 'POST',
|
||||
headers: {
|
||||
'Content-Type': 'application/json',
|
||||
'App-Key': _appKey,
|
||||
},
|
||||
credentials: 'omit',
|
||||
body: JSON.stringify({
|
||||
timestamp: new Date().toISOString(),
|
||||
sessionId: _sessionId,
|
||||
eventName: eventName,
|
||||
systemProps: {
|
||||
locale,
|
||||
isDebug: _isDebug,
|
||||
appVersion: _appVersion,
|
||||
sdkVersion: globalThis.__APTABASE_SDK_VERSION__ ?? `aptabase-web@${process.env.PKG_VERSION}`,
|
||||
},
|
||||
props: props,
|
||||
}),
|
||||
});
|
||||
|
||||
if (response.status >= 300) {
|
||||
const responseBody = await response.text();
|
||||
console.warn(`Failed to send event "${eventName}": ${response.status} ${responseBody}`);
|
||||
}
|
||||
} catch (e) {
|
||||
console.warn(`Failed to send event "${eventName}"`);
|
||||
console.warn(e);
|
||||
}
|
||||
}
|
||||
|
||||
function getBaseUrl(region: string, options?: AptabaseOptions): string | undefined {
|
||||
if (region === 'SH') {
|
||||
if (!options?.host) {
|
||||
console.warn(`Host parameter must be defined when using Self-Hosted App Key. Tracking will be disabled.`);
|
||||
return;
|
||||
}
|
||||
return options.host;
|
||||
}
|
||||
|
||||
return _hosts[region];
|
||||
}
|
||||
|
||||
function getBrowserLocale(): string | null {
|
||||
if (typeof navigator === 'undefined') {
|
||||
return null;
|
||||
}
|
||||
|
||||
if (navigator.languages.length > 0) {
|
||||
return navigator.languages[0];
|
||||
}
|
||||
|
||||
return navigator.language;
|
||||
}
|
||||
|
||||
function getIsDebug(): boolean {
|
||||
if (process.env.NODE_ENV === 'development') {
|
||||
return true;
|
||||
}
|
||||
|
||||
if (typeof location === 'undefined') {
|
||||
return false;
|
||||
}
|
||||
|
||||
return location.hostname === 'localhost';
|
||||
await sendEvent({
|
||||
sessionId,
|
||||
appKey: _appKey,
|
||||
isDebug: _options?.isDebug,
|
||||
appVersion: _options?.appVersion,
|
||||
sdkVersion,
|
||||
eventName,
|
||||
props,
|
||||
});
|
||||
}
|
||||
|
|
|
@ -1,8 +0,0 @@
|
|||
export function newSessionId(): string {
|
||||
const epochInSeconds = Math.floor(Date.now() / 1000).toString();
|
||||
const random = Math.floor(Math.random() * 100000000)
|
||||
.toString()
|
||||
.padStart(8, '0');
|
||||
|
||||
return epochInSeconds + random;
|
||||
}
|
Loading…
Add table
Add a link
Reference in a new issue