Strip down the entire project
This commit is contained in:
parent
a0a02458b0
commit
7416341746
95 changed files with 647 additions and 43115 deletions
|
@ -1,93 +0,0 @@
|
|||
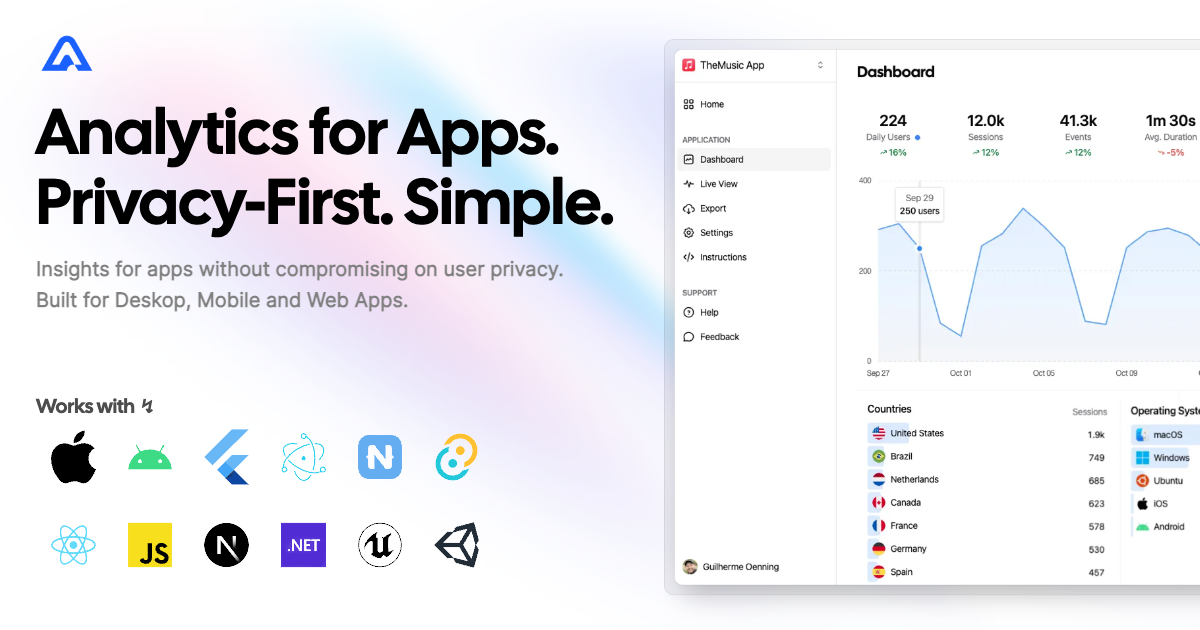
|
||||
|
||||
# Aptabase SDK for Angular Apps
|
||||
|
||||
A tiny SDK to instrument your Angular apps with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
## Setup
|
||||
|
||||
1. Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/angular
|
||||
```
|
||||
|
||||
2. Get your `App Key` from Aptabase, you can find it in the `Instructions` page.
|
||||
|
||||
3. Pass the `App Key` when initializing your app by importing a module or providing a function.
|
||||
|
||||
### Setup for Standalone API
|
||||
|
||||
Provide `provideAptabaseAnalytics` in the ApplicationConfig when bootstrapping.
|
||||
|
||||
```ts
|
||||
import { provideAptabaseAnalytics } from '@aptabase/angular';
|
||||
|
||||
bootstrapApplication(AppComponent, {
|
||||
providers: [..., provideAptabaseAnalytics('<YOUR_APP_KEY>')],
|
||||
}).catch((err) => console.error(err));
|
||||
```
|
||||
|
||||
[Full example here](examples/example-standalone/src/app)
|
||||
|
||||
### Setup for NgModules
|
||||
|
||||
Import `AptabaseAnalyticsModule` in your root AppModule.
|
||||
|
||||
```ts
|
||||
import { AptabaseAnalyticsModule } from '@aptabase/angular';
|
||||
|
||||
@NgModule({
|
||||
declarations: [AppComponent],
|
||||
imports: [..., AptabaseAnalyticsModule.forRoot('<YOUR_APP_KEY>')],
|
||||
providers: [],
|
||||
bootstrap: [AppComponent],
|
||||
})
|
||||
export class AppModule {}
|
||||
|
||||
```
|
||||
|
||||
[Full example here](examples/example-modules/src/app)
|
||||
|
||||
## Advanced setup
|
||||
|
||||
Both versions support also support an optional second parameter `AptabaseOptions` to pass in additional options.
|
||||
|
||||
```ts
|
||||
export type AptabaseOptions = {
|
||||
// Custom host for self-hosted Aptabase.
|
||||
host?: string;
|
||||
// Custom path for API endpoint. Useful when using reverse proxy.
|
||||
apiUrl?: string;
|
||||
// Defines the app version.
|
||||
appVersion?: string;
|
||||
// Defines whether the app is running on debug mode.
|
||||
isDebug?: boolean;
|
||||
};
|
||||
```
|
||||
|
||||
## Tracking Events with Aptabase
|
||||
|
||||
After the initial setup the `AptabaseAnalyticsService` can be used to start tracking events.
|
||||
Simply inject the service in a component to start tracking events:
|
||||
|
||||
```ts
|
||||
import { AptabaseAnalyticsService } from '@aptabase/angular';
|
||||
|
||||
export class AppComponent {
|
||||
constructor(private _analyticsService: AptabaseAnalyticsService) {}
|
||||
|
||||
increment() {
|
||||
this.counter++;
|
||||
this._analyticsService.trackEvent('increment');
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to subscribe to `trackEvent` function, it'll run in the background.
|
||||
4. Only strings and numeric values are allowed on custom properties.
|
|
@ -1,230 +0,0 @@
|
|||
{
|
||||
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
|
||||
"version": 1,
|
||||
"newProjectRoot": ".",
|
||||
"projects": {
|
||||
"aptabase-angular": {
|
||||
"projectType": "library",
|
||||
"root": "aptabase-angular",
|
||||
"sourceRoot": "aptabase-angular/src",
|
||||
"prefix": "lib",
|
||||
"architect": {
|
||||
"build": {
|
||||
"builder": "@angular-devkit/build-angular:ng-packagr",
|
||||
"options": {
|
||||
"project": "aptabase-angular/ng-package.json"
|
||||
},
|
||||
"configurations": {
|
||||
"production": {
|
||||
"tsConfig": "aptabase-angular/tsconfig.lib.prod.json"
|
||||
},
|
||||
"development": {
|
||||
"tsConfig": "aptabase-angular/tsconfig.lib.json"
|
||||
}
|
||||
},
|
||||
"defaultConfiguration": "production"
|
||||
},
|
||||
"test": {
|
||||
"builder": "@angular-devkit/build-angular:karma",
|
||||
"options": {
|
||||
"tsConfig": "aptabase-angular/tsconfig.spec.json",
|
||||
"polyfills": ["zone.js", "zone.js/testing"]
|
||||
}
|
||||
}
|
||||
}
|
||||
},
|
||||
"example-standalone": {
|
||||
"projectType": "application",
|
||||
"schematics": {
|
||||
"@schematics/angular:component": {
|
||||
"inlineTemplate": true,
|
||||
"inlineStyle": true,
|
||||
"style": "scss",
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:class": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:directive": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:guard": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:interceptor": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:pipe": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:resolver": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:service": {
|
||||
"skipTests": true
|
||||
}
|
||||
},
|
||||
"root": "examples/example-standalone",
|
||||
"sourceRoot": "examples/example-standalone/src",
|
||||
"prefix": "app",
|
||||
"architect": {
|
||||
"build": {
|
||||
"builder": "@angular-devkit/build-angular:application",
|
||||
"options": {
|
||||
"outputPath": "dist/example-standalone",
|
||||
"index": "examples/example-standalone/src/index.html",
|
||||
"browser": "examples/example-standalone/src/main.ts",
|
||||
"polyfills": ["zone.js"],
|
||||
"tsConfig": "examples/example-standalone/tsconfig.app.json",
|
||||
"inlineStyleLanguage": "scss",
|
||||
"assets": ["examples/example-standalone/src/favicon.ico", "examples/example-standalone/src/assets"],
|
||||
"styles": ["examples/example-standalone/src/styles.scss"],
|
||||
"scripts": []
|
||||
},
|
||||
"configurations": {
|
||||
"production": {
|
||||
"budgets": [
|
||||
{
|
||||
"type": "initial",
|
||||
"maximumWarning": "500kb",
|
||||
"maximumError": "1mb"
|
||||
},
|
||||
{
|
||||
"type": "anyComponentStyle",
|
||||
"maximumWarning": "2kb",
|
||||
"maximumError": "4kb"
|
||||
}
|
||||
],
|
||||
"outputHashing": "all"
|
||||
},
|
||||
"development": {
|
||||
"optimization": false,
|
||||
"extractLicenses": false,
|
||||
"sourceMap": true
|
||||
}
|
||||
},
|
||||
"defaultConfiguration": "production"
|
||||
},
|
||||
"serve": {
|
||||
"builder": "@angular-devkit/build-angular:dev-server",
|
||||
"configurations": {
|
||||
"production": {
|
||||
"buildTarget": "example-standalone:build:production"
|
||||
},
|
||||
"development": {
|
||||
"buildTarget": "example-standalone:build:development"
|
||||
}
|
||||
},
|
||||
"options": {
|
||||
"ssl": true
|
||||
},
|
||||
"defaultConfiguration": "development"
|
||||
},
|
||||
"extract-i18n": {
|
||||
"builder": "@angular-devkit/build-angular:extract-i18n",
|
||||
"options": {
|
||||
"buildTarget": "example-standalone:build"
|
||||
}
|
||||
}
|
||||
}
|
||||
},
|
||||
"example-modules": {
|
||||
"projectType": "application",
|
||||
"schematics": {
|
||||
"@schematics/angular:component": {
|
||||
"inlineTemplate": true,
|
||||
"inlineStyle": true,
|
||||
"style": "scss",
|
||||
"skipTests": true,
|
||||
"standalone": false
|
||||
},
|
||||
"@schematics/angular:class": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:directive": {
|
||||
"skipTests": true,
|
||||
"standalone": false
|
||||
},
|
||||
"@schematics/angular:guard": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:interceptor": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:pipe": {
|
||||
"skipTests": true,
|
||||
"standalone": false
|
||||
},
|
||||
"@schematics/angular:resolver": {
|
||||
"skipTests": true
|
||||
},
|
||||
"@schematics/angular:service": {
|
||||
"skipTests": true
|
||||
}
|
||||
},
|
||||
"root": "examples/example-modules",
|
||||
"sourceRoot": "examples/example-modules/src",
|
||||
"prefix": "app",
|
||||
"architect": {
|
||||
"build": {
|
||||
"builder": "@angular-devkit/build-angular:application",
|
||||
"options": {
|
||||
"outputPath": "dist/example-modules",
|
||||
"index": "examples/example-modules/src/index.html",
|
||||
"browser": "examples/example-modules/src/main.ts",
|
||||
"polyfills": ["zone.js"],
|
||||
"tsConfig": "examples/example-modules/tsconfig.app.json",
|
||||
"inlineStyleLanguage": "scss",
|
||||
"assets": ["examples/example-modules/src/favicon.ico", "examples/example-modules/src/assets"],
|
||||
"styles": ["examples/example-modules/src/styles.scss"],
|
||||
"scripts": []
|
||||
},
|
||||
"configurations": {
|
||||
"production": {
|
||||
"budgets": [
|
||||
{
|
||||
"type": "initial",
|
||||
"maximumWarning": "500kb",
|
||||
"maximumError": "1mb"
|
||||
},
|
||||
{
|
||||
"type": "anyComponentStyle",
|
||||
"maximumWarning": "2kb",
|
||||
"maximumError": "4kb"
|
||||
}
|
||||
],
|
||||
"outputHashing": "all"
|
||||
},
|
||||
"development": {
|
||||
"optimization": false,
|
||||
"extractLicenses": false,
|
||||
"sourceMap": true
|
||||
}
|
||||
},
|
||||
"defaultConfiguration": "production"
|
||||
},
|
||||
"serve": {
|
||||
"builder": "@angular-devkit/build-angular:dev-server",
|
||||
"configurations": {
|
||||
"production": {
|
||||
"buildTarget": "example-modules:build:production"
|
||||
},
|
||||
"development": {
|
||||
"buildTarget": "example-modules:build:development"
|
||||
}
|
||||
},
|
||||
"options": {
|
||||
"ssl": true
|
||||
},
|
||||
"defaultConfiguration": "development"
|
||||
},
|
||||
"extract-i18n": {
|
||||
"builder": "@angular-devkit/build-angular:extract-i18n",
|
||||
"options": {
|
||||
"buildTarget": "example-modules:build"
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
|
@ -1,21 +0,0 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2023 Sumbit Labs Ltd.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -1,93 +0,0 @@
|
|||
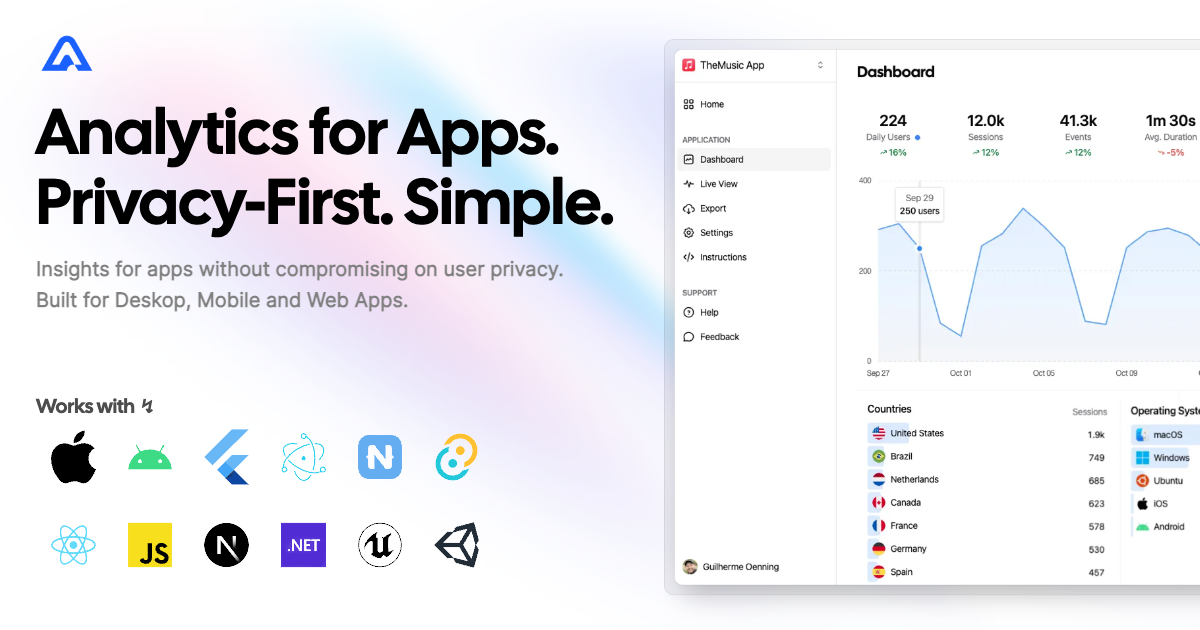
|
||||
|
||||
# Aptabase SDK for Angular Apps
|
||||
|
||||
A tiny SDK to instrument your Angular apps with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
## Setup
|
||||
|
||||
1. Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/angular
|
||||
```
|
||||
|
||||
2. Get your `App Key` from Aptabase, you can find it in the `Instructions` page.
|
||||
|
||||
3. Pass the `App Key` when initializing your app by importing a module or providing a function.
|
||||
|
||||
### Setup for Standalone API
|
||||
|
||||
Provide `provideAptabaseAnalytics` in the ApplicationConfig when bootstrapping.
|
||||
|
||||
```ts
|
||||
import { provideAptabaseAnalytics } from '@aptabase/angular';
|
||||
|
||||
bootstrapApplication(AppComponent, {
|
||||
providers: [..., provideAptabaseAnalytics('<YOUR_APP_KEY>')],
|
||||
}).catch((err) => console.error(err));
|
||||
```
|
||||
|
||||
[Full example here](../examples/example-standalone/src/app)
|
||||
|
||||
### Setup for NgModules
|
||||
|
||||
Import `AptabaseAnalyticsModule` in your root AppModule.
|
||||
|
||||
```ts
|
||||
import { AptabaseAnalyticsModule } from '@aptabase/angular';
|
||||
|
||||
@NgModule({
|
||||
declarations: [AppComponent],
|
||||
imports: [..., AptabaseAnalyticsModule.forRoot('<YOUR_APP_KEY>')],
|
||||
providers: [],
|
||||
bootstrap: [AppComponent],
|
||||
})
|
||||
export class AppModule {}
|
||||
|
||||
```
|
||||
|
||||
[Full example here](../examples/example-modules/src/app)
|
||||
|
||||
## Advanced setup
|
||||
|
||||
Both versions support also support an optional second parameter `AptabaseOptions` to pass in additional options.
|
||||
|
||||
```ts
|
||||
export type AptabaseOptions = {
|
||||
// Custom host for self-hosted Aptabase.
|
||||
host?: string;
|
||||
// Custom path for API endpoint. Useful when using reverse proxy.
|
||||
apiUrl?: string;
|
||||
// Defines the app version.
|
||||
appVersion?: string;
|
||||
// Defines whether the app is running on debug mode.
|
||||
isDebug?: boolean;
|
||||
};
|
||||
```
|
||||
|
||||
## Tracking Events with Aptabase
|
||||
|
||||
After the initial setup the `AptabaseAnalyticsService` can be used to start tracking events.
|
||||
Simply inject the service in a component to start tracking events:
|
||||
|
||||
```ts
|
||||
import { AptabaseAnalyticsService } from '@aptabase/angular';
|
||||
|
||||
export class AppComponent {
|
||||
constructor(private _analyticsService: AptabaseAnalyticsService) {}
|
||||
|
||||
increment() {
|
||||
this.counter++;
|
||||
this._analyticsService.trackEvent('increment');
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to subscribe to `trackEvent` function, it'll run in the background.
|
||||
4. Only strings and numeric values are allowed on custom properties.
|
|
@ -1,7 +0,0 @@
|
|||
{
|
||||
"$schema": "../node_modules/ng-packagr/ng-package.schema.json",
|
||||
"dest": "dist",
|
||||
"lib": {
|
||||
"entryFile": "src/public-api.ts"
|
||||
}
|
||||
}
|
|
@ -1,28 +0,0 @@
|
|||
{
|
||||
"name": "@aptabase/angular",
|
||||
"version": "0.0.6",
|
||||
"description": "Angular SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/aptabase/aptabase-js.git",
|
||||
"directory": "packages/angular/aptabase-angular"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/aptabase/aptabase-js/issues"
|
||||
},
|
||||
"homepage": "https://github.com/aptabase/aptabase-js",
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"pre-build": "cp ../../shared.ts ./src",
|
||||
"build": "npm run pre-build && ng build aptabase-angular",
|
||||
"prepublishOnly": "npm run build"
|
||||
},
|
||||
"peerDependencies": {
|
||||
"@angular/common": "^17.0.0",
|
||||
"@angular/core": "^17.0.0"
|
||||
},
|
||||
"dependencies": {
|
||||
"tslib": "^2.3.0"
|
||||
},
|
||||
"sideEffects": false
|
||||
}
|
|
@ -1,37 +0,0 @@
|
|||
import { AptabaseOptions, getApiUrl, inMemorySessionId, sendEvent, validateAppKey } from '../shared';
|
||||
|
||||
// Session expires after 1 hour of inactivity
|
||||
// TODO move this to shared?
|
||||
const SESSION_TIMEOUT = 1 * 60 * 60;
|
||||
const pkgVersion = '0.0.0'; // bog: TODO fix this version
|
||||
const sdkVersion = `aptabase-angular@${pkgVersion}`;
|
||||
|
||||
export class AptabaseAnalyticsService {
|
||||
private _apiUrl: string | undefined;
|
||||
|
||||
constructor(
|
||||
private _appKey: string,
|
||||
private _options: AptabaseOptions,
|
||||
) {
|
||||
if (!validateAppKey(this._appKey)) return;
|
||||
|
||||
this._apiUrl = this._options.apiUrl ?? getApiUrl(this._appKey, this._options);
|
||||
}
|
||||
|
||||
async trackEvent(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
if (!this._apiUrl) return;
|
||||
|
||||
const sessionId = inMemorySessionId(SESSION_TIMEOUT);
|
||||
|
||||
await sendEvent({
|
||||
apiUrl: this._apiUrl,
|
||||
sessionId,
|
||||
appKey: this._appKey,
|
||||
isDebug: this._options?.isDebug,
|
||||
appVersion: this._options?.appVersion,
|
||||
sdkVersion,
|
||||
eventName,
|
||||
props,
|
||||
});
|
||||
}
|
||||
}
|
|
@ -1,28 +0,0 @@
|
|||
import { EnvironmentProviders, NgModule, makeEnvironmentProviders } from '@angular/core';
|
||||
|
||||
import { AptabaseOptions } from '../shared';
|
||||
import { AptabaseAnalyticsService } from './analytics.service';
|
||||
|
||||
export function provideAptabaseAnalytics(appKey: string, options: AptabaseOptions = {}): EnvironmentProviders {
|
||||
return makeEnvironmentProviders([
|
||||
{
|
||||
provide: AptabaseAnalyticsService,
|
||||
useValue: new AptabaseAnalyticsService(appKey, options),
|
||||
},
|
||||
]);
|
||||
}
|
||||
|
||||
@NgModule()
|
||||
export class AptabaseAnalyticsModule {
|
||||
static forRoot(appKey: string, options: AptabaseOptions = {}) {
|
||||
return {
|
||||
ngModule: AptabaseAnalyticsModule,
|
||||
providers: [
|
||||
{
|
||||
provide: AptabaseAnalyticsService,
|
||||
useValue: new AptabaseAnalyticsService(appKey, options),
|
||||
},
|
||||
],
|
||||
};
|
||||
}
|
||||
}
|
|
@ -1,6 +0,0 @@
|
|||
/*
|
||||
* Public API Surface of aptabase-angular
|
||||
*/
|
||||
|
||||
export * from './lib/analytics.service';
|
||||
export * from './lib/index';
|
|
@ -1,11 +0,0 @@
|
|||
/* To learn more about this file see: https://angular.io/config/tsconfig. */
|
||||
{
|
||||
"extends": "../tsconfig.json",
|
||||
"compilerOptions": {
|
||||
"outDir": "../out-tsc/lib",
|
||||
"declaration": true,
|
||||
"declarationMap": true,
|
||||
"inlineSources": true
|
||||
},
|
||||
"exclude": ["**/*.spec.ts"]
|
||||
}
|
|
@ -1,10 +0,0 @@
|
|||
/* To learn more about this file see: https://angular.io/config/tsconfig. */
|
||||
{
|
||||
"extends": "./tsconfig.lib.json",
|
||||
"compilerOptions": {
|
||||
"declarationMap": false
|
||||
},
|
||||
"angularCompilerOptions": {
|
||||
"compilationMode": "partial"
|
||||
}
|
||||
}
|
|
@ -1,14 +0,0 @@
|
|||
/* To learn more about this file see: https://angular.io/config/tsconfig. */
|
||||
{
|
||||
"extends": "../tsconfig.json",
|
||||
"compilerOptions": {
|
||||
"outDir": "../out-tsc/spec",
|
||||
"types": [
|
||||
"jasmine"
|
||||
]
|
||||
},
|
||||
"include": [
|
||||
"**/*.spec.ts",
|
||||
"**/*.d.ts"
|
||||
]
|
||||
}
|
|
@ -1,10 +0,0 @@
|
|||
import { NgModule } from '@angular/core';
|
||||
import { RouterModule, Routes } from '@angular/router';
|
||||
|
||||
const routes: Routes = [];
|
||||
|
||||
@NgModule({
|
||||
imports: [RouterModule.forRoot(routes)],
|
||||
exports: [RouterModule]
|
||||
})
|
||||
export class AppRoutingModule { }
|
|
@ -1,23 +0,0 @@
|
|||
import { Component } from '@angular/core';
|
||||
import { AptabaseAnalyticsService } from '@aptabase/angular';
|
||||
|
||||
@Component({
|
||||
selector: 'app-root',
|
||||
template: `
|
||||
<h1>Welcome to {{ title }}!</h1>
|
||||
|
||||
<button (click)="trackButtonClick()">Track event</button>
|
||||
|
||||
<router-outlet />
|
||||
`,
|
||||
styles: [],
|
||||
})
|
||||
export class AppComponent {
|
||||
constructor(private _analyticsService: AptabaseAnalyticsService) {}
|
||||
|
||||
title = 'example-modules';
|
||||
|
||||
trackButtonClick() {
|
||||
this._analyticsService.trackEvent('module_btn_click');
|
||||
}
|
||||
}
|
|
@ -1,14 +0,0 @@
|
|||
import { NgModule } from '@angular/core';
|
||||
import { BrowserModule } from '@angular/platform-browser';
|
||||
|
||||
import { AptabaseAnalyticsModule } from '@aptabase/angular';
|
||||
import { AppRoutingModule } from './app-routing.module';
|
||||
import { AppComponent } from './app.component';
|
||||
|
||||
@NgModule({
|
||||
declarations: [AppComponent],
|
||||
imports: [BrowserModule, AppRoutingModule, AptabaseAnalyticsModule.forRoot('A-EU-1280395555')],
|
||||
providers: [],
|
||||
bootstrap: [AppComponent],
|
||||
})
|
||||
export class AppModule {}
|
Binary file not shown.
Before Width: | Height: | Size: 15 KiB |
|
@ -1,13 +0,0 @@
|
|||
<!doctype html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="utf-8">
|
||||
<title>ExampleModules</title>
|
||||
<base href="/">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1">
|
||||
<link rel="icon" type="image/x-icon" href="favicon.ico">
|
||||
</head>
|
||||
<body>
|
||||
<app-root></app-root>
|
||||
</body>
|
||||
</html>
|
|
@ -1,7 +0,0 @@
|
|||
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
|
||||
|
||||
import { AppModule } from './app/app.module';
|
||||
|
||||
|
||||
platformBrowserDynamic().bootstrapModule(AppModule)
|
||||
.catch(err => console.error(err));
|
|
@ -1 +0,0 @@
|
|||
/* You can add global styles to this file, and also import other style files */
|
|
@ -1,9 +0,0 @@
|
|||
/* To learn more about this file see: https://angular.io/config/tsconfig. */
|
||||
{
|
||||
"extends": "../../tsconfig.json",
|
||||
"compilerOptions": {
|
||||
"outDir": "../../out-tsc/app"
|
||||
},
|
||||
"files": ["src/main.ts"],
|
||||
"include": ["src/**/*.d.ts"]
|
||||
}
|
|
@ -1,26 +0,0 @@
|
|||
import { Component, inject } from '@angular/core';
|
||||
import { RouterOutlet } from '@angular/router';
|
||||
import { AptabaseAnalyticsService } from '@aptabase/angular';
|
||||
|
||||
@Component({
|
||||
selector: 'app-root',
|
||||
standalone: true,
|
||||
imports: [RouterOutlet],
|
||||
template: `
|
||||
<h1>Welcome to {{ title }}!</h1>
|
||||
|
||||
<button (click)="trackButtonClick()">Track event</button>
|
||||
|
||||
<router-outlet />
|
||||
`,
|
||||
styles: [],
|
||||
})
|
||||
export class AppComponent {
|
||||
private _analytics = inject(AptabaseAnalyticsService);
|
||||
|
||||
title = 'example-standalone';
|
||||
|
||||
trackButtonClick() {
|
||||
this._analytics.trackEvent('home_btn_click', { customProp: 'Hello from Aptabase' });
|
||||
}
|
||||
}
|
|
@ -1,9 +0,0 @@
|
|||
import { ApplicationConfig } from '@angular/core';
|
||||
import { provideRouter } from '@angular/router';
|
||||
import { provideAptabaseAnalytics } from '@aptabase/angular';
|
||||
|
||||
import { routes } from './app.routes';
|
||||
|
||||
export const appConfig: ApplicationConfig = {
|
||||
providers: [provideRouter(routes), provideAptabaseAnalytics('A-DEV-1624170742')],
|
||||
};
|
|
@ -1,3 +0,0 @@
|
|||
import { Routes } from '@angular/router';
|
||||
|
||||
export const routes: Routes = [];
|
Binary file not shown.
Before Width: | Height: | Size: 15 KiB |
|
@ -1,13 +0,0 @@
|
|||
<!doctype html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="utf-8">
|
||||
<title>ExampleStandalone</title>
|
||||
<base href="/">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1">
|
||||
<link rel="icon" type="image/x-icon" href="favicon.ico">
|
||||
</head>
|
||||
<body>
|
||||
<app-root></app-root>
|
||||
</body>
|
||||
</html>
|
|
@ -1,5 +0,0 @@
|
|||
import { bootstrapApplication } from '@angular/platform-browser';
|
||||
import { AppComponent } from './app/app.component';
|
||||
import { appConfig } from './app/app.config';
|
||||
|
||||
bootstrapApplication(AppComponent, appConfig).catch((err) => console.error(err));
|
|
@ -1 +0,0 @@
|
|||
/* You can add global styles to this file, and also import other style files */
|
|
@ -1,9 +0,0 @@
|
|||
/* To learn more about this file see: https://angular.io/config/tsconfig. */
|
||||
{
|
||||
"extends": "../../tsconfig.json",
|
||||
"compilerOptions": {
|
||||
"outDir": "../../out-tsc/app"
|
||||
},
|
||||
"files": ["src/main.ts"],
|
||||
"include": ["src/**/*.d.ts"]
|
||||
}
|
12758
packages/angular/package-lock.json
generated
12758
packages/angular/package-lock.json
generated
File diff suppressed because it is too large
Load diff
|
@ -1,43 +0,0 @@
|
|||
{
|
||||
"name": "@aptabase/angular",
|
||||
"private": true,
|
||||
"version": "0.0.2",
|
||||
"description": "Angular SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"scripts": {
|
||||
"ng": "ng",
|
||||
"start": "ng serve example-standalone",
|
||||
"start-modules-sample": "ng serve example-modules",
|
||||
"pre-build": "cp ../shared.ts ./aptabase-angular/src",
|
||||
"build": "npm run pre-build && ng build aptabase-angular",
|
||||
"watch": "ng build --watch --configuration development",
|
||||
"test": "ng test"
|
||||
},
|
||||
"dependencies": {
|
||||
"@angular/animations": "^17.0.0",
|
||||
"@angular/common": "^17.0.0",
|
||||
"@angular/compiler": "^17.0.0",
|
||||
"@angular/core": "^17.0.0",
|
||||
"@angular/forms": "^17.0.0",
|
||||
"@angular/platform-browser": "^17.0.0",
|
||||
"@angular/platform-browser-dynamic": "^17.0.0",
|
||||
"@angular/router": "^17.0.0",
|
||||
"rxjs": "~7.8.0",
|
||||
"tslib": "^2.3.0",
|
||||
"zone.js": "~0.14.2"
|
||||
},
|
||||
"devDependencies": {
|
||||
"@angular-devkit/build-angular": "^17.3.7",
|
||||
"@angular/cli": "^17.0.8",
|
||||
"@angular/compiler-cli": "^17.0.0",
|
||||
"@types/jasmine": "~5.1.0",
|
||||
"@types/node": "20.5.7",
|
||||
"jasmine-core": "~5.1.0",
|
||||
"karma": "~6.4.0",
|
||||
"karma-chrome-launcher": "~3.2.0",
|
||||
"karma-coverage": "~2.2.0",
|
||||
"karma-jasmine": "~5.1.0",
|
||||
"karma-jasmine-html-reporter": "~2.1.0",
|
||||
"ng-packagr": "^17.3.0",
|
||||
"typescript": "~5.2.2"
|
||||
}
|
||||
}
|
|
@ -1,36 +0,0 @@
|
|||
/* To learn more about this file see: https://angular.io/config/tsconfig. */
|
||||
{
|
||||
"compileOnSave": false,
|
||||
"include": ["**/*.ts", "../shared/*.ts"],
|
||||
"compilerOptions": {
|
||||
"paths": {
|
||||
"@aptabase/angular": ["./aptabase-angular/src/public-api.ts"],
|
||||
"@aptabase/angular/*": ["./aptabase-angular/src/*"]
|
||||
},
|
||||
"outDir": "./dist/out-tsc",
|
||||
"forceConsistentCasingInFileNames": true,
|
||||
"strict": true,
|
||||
"noImplicitOverride": true,
|
||||
"noPropertyAccessFromIndexSignature": true,
|
||||
"noImplicitReturns": true,
|
||||
"noFallthroughCasesInSwitch": true,
|
||||
"skipLibCheck": true,
|
||||
"esModuleInterop": true,
|
||||
"sourceMap": true,
|
||||
"declaration": false,
|
||||
"experimentalDecorators": true,
|
||||
"moduleResolution": "node",
|
||||
"importHelpers": true,
|
||||
"target": "ES2022",
|
||||
"module": "ES2022",
|
||||
"useDefineForClassFields": false,
|
||||
"lib": ["ES2022", "dom"],
|
||||
"types": ["node", "chrome"]
|
||||
},
|
||||
"angularCompilerOptions": {
|
||||
"enableI18nLegacyMessageIdFormat": false,
|
||||
"strictInjectionParameters": true,
|
||||
"strictInputAccessModifiers": true,
|
||||
"strictTemplates": true
|
||||
}
|
||||
}
|
|
@ -1,12 +0,0 @@
|
|||
## 0.1.2
|
||||
|
||||
- Move browser locale and is debug reads from import to first event to better support SSR
|
||||
|
||||
## 0.1.1
|
||||
|
||||
- Support for custom API path
|
||||
- use `installType` to determine if the extension is in debug mode
|
||||
|
||||
## 0.1.0
|
||||
|
||||
- Initial version of the Aptabase SDK for Browser Extensions
|
|
@ -1,21 +0,0 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2023 Sumbit Labs Ltd.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -1,44 +0,0 @@
|
|||
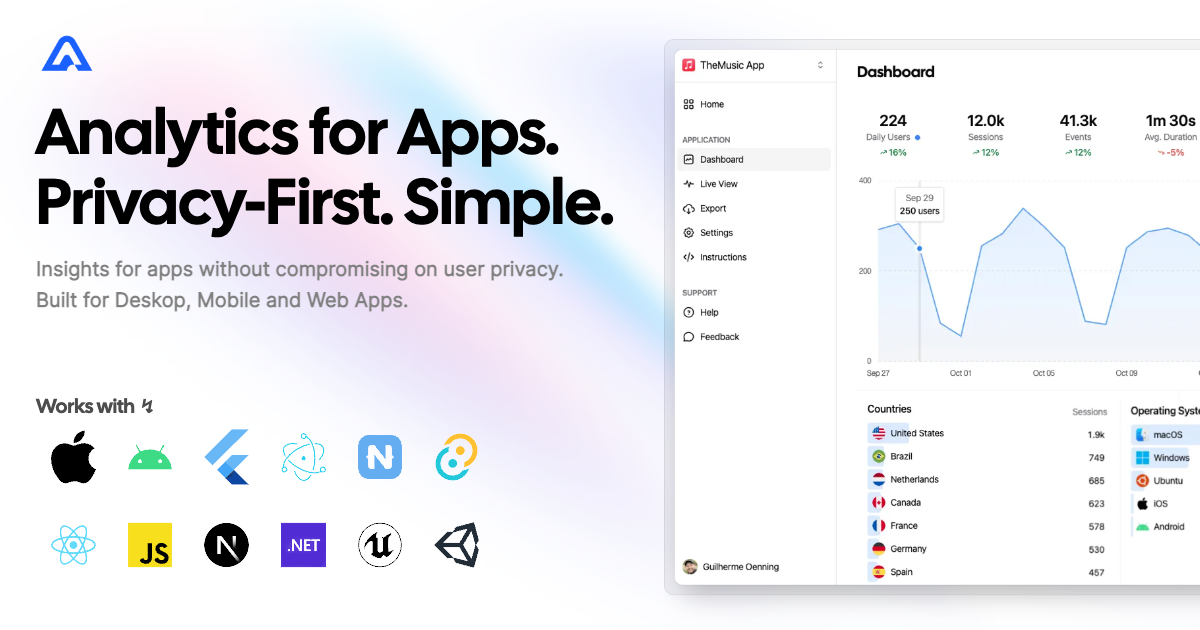
|
||||
|
||||
# Aptabase SDK for Browser Extensions
|
||||
|
||||
A tiny SDK (1 kB) to instrument your Browser/Chrome extensions with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
## Install
|
||||
|
||||
Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/browser
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
First you need to get your `App Key` from Aptabase, you can find it in the `Instructions` menu on the left side menu.
|
||||
|
||||
Initialize the SDK in your `Background Script` using your `App Key`:
|
||||
|
||||
```js
|
||||
import { init } from '@aptabase/browser';
|
||||
|
||||
init('<YOUR_APP_KEY>'); // 👈 this is where you enter your App Key
|
||||
```
|
||||
|
||||
The `init` function also supports an optional second parameter, which is an object with the `isDebug`property. Your data is segregated between development and production environments, so it's important to set this value correctly. By default the SDK will check if the extension was installed from an Extension Store, and if it wasn't it'll assume it's in development mode. If you want to override this behavior, you can set the`isDebug` property.
|
||||
|
||||
Afterwards you can start tracking events with `trackEvent` from anywhere in your extension:
|
||||
|
||||
```js
|
||||
import { trackEvent } from '@aptabase/browser';
|
||||
|
||||
trackEvent('connect_click'); // An event with no properties
|
||||
trackEvent('play_music', { name: 'Here comes the sun' }); // An event with a custom property
|
||||
```
|
||||
|
||||
A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS, the app version, and other things.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to await the `trackEvent` function, it'll run in the background.
|
||||
4. Only strings and numeric values are allowed on custom properties
|
|
@ -1,36 +0,0 @@
|
|||
{
|
||||
"name": "@aptabase/browser",
|
||||
"version": "0.1.2",
|
||||
"type": "module",
|
||||
"description": "Browser Extension SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"main": "./dist/index.cjs",
|
||||
"module": "./dist/index.js",
|
||||
"types": "./dist/index.d.ts",
|
||||
"exports": {
|
||||
".": {
|
||||
"require": "./dist/index.cjs",
|
||||
"import": "./dist/index.js",
|
||||
"types": "./dist/index.d.ts"
|
||||
}
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/aptabase/aptabase-js.git",
|
||||
"directory": "packages/browser"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/aptabase/aptabase-js/issues"
|
||||
},
|
||||
"homepage": "https://github.com/aptabase/aptabase-js",
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"build": "tsup",
|
||||
"prepublishOnly": "npm run build"
|
||||
},
|
||||
"files": [
|
||||
"README.md",
|
||||
"LICENSE",
|
||||
"dist",
|
||||
"package.json"
|
||||
]
|
||||
}
|
4
packages/browser/src/global.d.ts
vendored
4
packages/browser/src/global.d.ts
vendored
|
@ -1,4 +0,0 @@
|
|||
declare var aptabase: {
|
||||
init: Function;
|
||||
trackEvent: Function;
|
||||
};
|
|
@ -1,91 +0,0 @@
|
|||
import { getApiUrl, newSessionId, sendEvent, validateAppKey, type AptabaseOptions } from '../../shared';
|
||||
|
||||
// Session expires after 1 hour of inactivity
|
||||
const SESSION_TIMEOUT = 1 * 60 * 60;
|
||||
const sdkVersion = `aptabase-browser@${process.env.PKG_VERSION}`;
|
||||
const isWebContext = 'document' in globalThis;
|
||||
|
||||
let _appKey = '';
|
||||
let _apiUrl: string | undefined;
|
||||
let _options: AptabaseOptions | undefined;
|
||||
|
||||
globalThis.aptabase = {
|
||||
init,
|
||||
trackEvent,
|
||||
};
|
||||
|
||||
export async function init(appKey: string, options?: AptabaseOptions): Promise<void> {
|
||||
if (isWebContext) {
|
||||
console.error('@aptabase/browser can only be initialized in your background script.');
|
||||
return;
|
||||
}
|
||||
|
||||
if (!validateAppKey(appKey)) return;
|
||||
|
||||
const ext = await chrome.management.getSelf();
|
||||
|
||||
const isDebug = options?.isDebug ?? ext.installType === 'development';
|
||||
const appVersion = options?.appVersion ?? chrome.runtime.getManifest().version;
|
||||
|
||||
_apiUrl = options?.apiUrl ?? getApiUrl(appKey, options);
|
||||
_appKey = appKey;
|
||||
_options = { ...options, isDebug, appVersion };
|
||||
|
||||
chrome.runtime.onMessage.addListener((message, sender, sendResponse) => {
|
||||
if (message && message.type === '__aptabase__trackEvent') {
|
||||
trackEvent(message.eventName, message.props);
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
export async function trackEvent(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
if (isWebContext) {
|
||||
return chrome.runtime.sendMessage({ type: '__aptabase__trackEvent', eventName, props });
|
||||
}
|
||||
|
||||
if (!_apiUrl) return;
|
||||
const sessionId = await getSessionId();
|
||||
|
||||
return sendEvent({
|
||||
apiUrl: _apiUrl,
|
||||
sessionId,
|
||||
appKey: _appKey,
|
||||
isDebug: _options?.isDebug,
|
||||
appVersion: _options?.appVersion,
|
||||
sdkVersion,
|
||||
eventName,
|
||||
props,
|
||||
});
|
||||
}
|
||||
|
||||
type CachedItem = {
|
||||
id: string;
|
||||
lastTouched: number;
|
||||
};
|
||||
|
||||
let _sessionId = newSessionId();
|
||||
|
||||
async function getSessionId(): Promise<string> {
|
||||
const now = Date.now();
|
||||
|
||||
// If storage is not available, return the in memory session id
|
||||
// This id will be recreated if the extension is reloaded or the service worker becomes inactive
|
||||
if (!chrome?.storage?.local) return _sessionId;
|
||||
|
||||
return new Promise((resolve) => {
|
||||
chrome.storage.local.get('_ab_session_id', (result) => {
|
||||
let item = (result['_ab_session_id'] as CachedItem) ?? { id: _sessionId, lastTouched: Date.now() };
|
||||
|
||||
const diffInMs = now - item.lastTouched;
|
||||
const diffInSec = Math.floor(diffInMs / 1000);
|
||||
if (diffInSec > SESSION_TIMEOUT) {
|
||||
item.id = newSessionId();
|
||||
}
|
||||
item.lastTouched = now;
|
||||
|
||||
chrome.storage.local.set({ _ab_session_id: item }, () => {
|
||||
resolve(item.id);
|
||||
});
|
||||
});
|
||||
});
|
||||
}
|
|
@ -1,15 +0,0 @@
|
|||
{
|
||||
"compilerOptions": {
|
||||
"target": "es6",
|
||||
"strict": true,
|
||||
"moduleResolution": "node",
|
||||
"esModuleInterop": true,
|
||||
"jsx": "react-jsx",
|
||||
"baseUrl": ".",
|
||||
"paths": {
|
||||
"types": ["@types"]
|
||||
},
|
||||
"declaration": true,
|
||||
"declarationDir": "./dist"
|
||||
}
|
||||
}
|
|
@ -1,15 +0,0 @@
|
|||
import { defineConfig } from 'tsup';
|
||||
const { version } = require('./package.json');
|
||||
|
||||
export default defineConfig({
|
||||
entry: ['src/index.ts'],
|
||||
format: ['cjs', 'esm'],
|
||||
dts: true,
|
||||
splitting: false,
|
||||
minify: true,
|
||||
sourcemap: true,
|
||||
clean: true,
|
||||
env: {
|
||||
PKG_VERSION: version,
|
||||
},
|
||||
});
|
|
@ -1,49 +0,0 @@
|
|||
## 0.3.5
|
||||
|
||||
### Patch Changes
|
||||
|
||||
- 7ea8c11: fix: events tracked at page load are sometimes not sent
|
||||
|
||||
## 0.3.4
|
||||
|
||||
- Move browser locale and is debug reads from import to first event to better support SSR
|
||||
|
||||
## 0.3.3
|
||||
|
||||
- Rename `apiPath` to `apiUrl`
|
||||
|
||||
## 0.3.2
|
||||
|
||||
- Fix error when running on chrome
|
||||
|
||||
## 0.3.1
|
||||
|
||||
- Support for custom API path
|
||||
|
||||
## 0.3.0
|
||||
|
||||
- Internal refactor
|
||||
|
||||
## 0.2.2
|
||||
|
||||
- Updated dependencies
|
||||
|
||||
## 0.2.1
|
||||
|
||||
- Updated dependencies
|
||||
|
||||
## 0.2.0
|
||||
|
||||
- Updated dependencies
|
||||
|
||||
## 0.1.2
|
||||
|
||||
- Fixed an issue with client-side checking
|
||||
|
||||
## 0.1.1
|
||||
|
||||
- Remove warning log from Server Side Rendering
|
||||
|
||||
## 0.1.0
|
||||
|
||||
- Initial version of the React SDK for Aptabase
|
|
@ -1,21 +0,0 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2023 Sumbit Labs Ltd.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
|
@ -1,154 +0,0 @@
|
|||
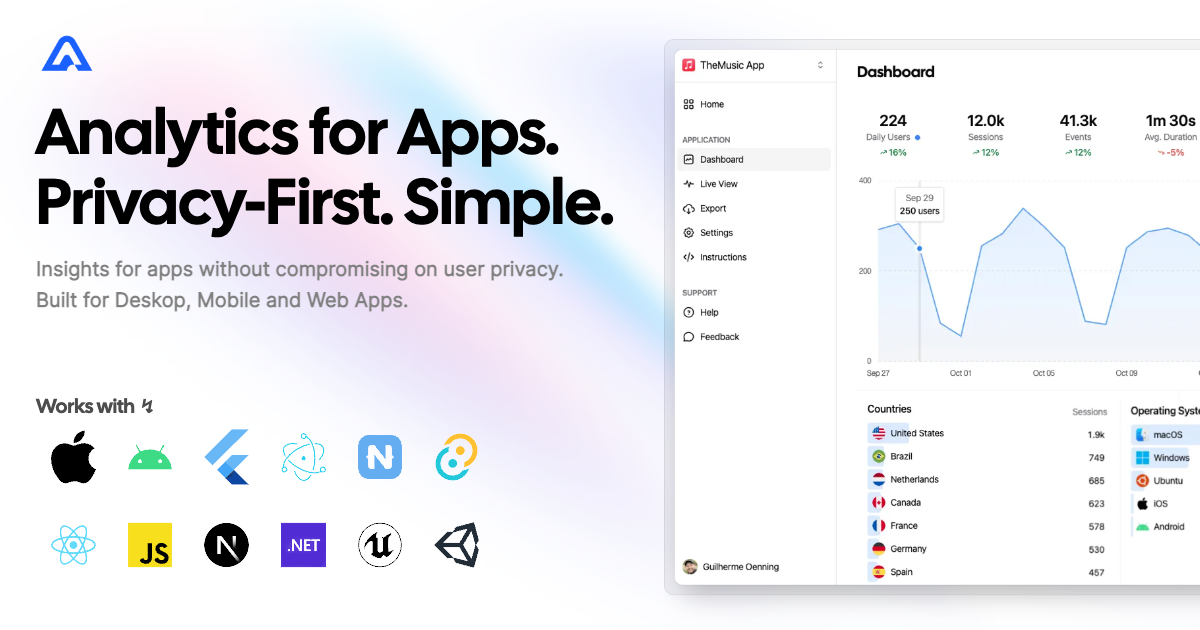
|
||||
|
||||
# Aptabase SDK for React Apps
|
||||
|
||||
A tiny SDK (1 kB) to instrument your React apps with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
## Setup
|
||||
|
||||
1. Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/react
|
||||
```
|
||||
|
||||
2. Get your `App Key` from Aptabase, you can find it in the `Instructions` menu on the left side menu.
|
||||
|
||||
3. Initialize the `AptabaseProvider` component to your app based on your framework.
|
||||
|
||||
<details>
|
||||
<summary>Setup for Next.js (App Router)</summary>
|
||||
|
||||
Add `AptabaseProvider` to your RootLayout component:
|
||||
|
||||
```tsx
|
||||
import { AptabaseProvider } from '@aptabase/react';
|
||||
|
||||
export default function RootLayout({ children }: { children: React.ReactNode }) {
|
||||
return (
|
||||
<html lang="en">
|
||||
<body>
|
||||
<AptabaseProvider appKey="<YOUR_APP_KEY>">{children}</AptabaseProvider>
|
||||
</body>
|
||||
</html>
|
||||
);
|
||||
}
|
||||
```
|
||||
|
||||
</details>
|
||||
|
||||
<details>
|
||||
<summary>Setup for Next.js (Pages Router)</summary>
|
||||
|
||||
Add `AptabaseProvider` to your `_app` component:
|
||||
|
||||
```tsx
|
||||
import { AptabaseProvider } from '@aptabase/react';
|
||||
import type { AppProps } from 'next/app';
|
||||
|
||||
export default function App({ Component, pageProps }: AppProps) {
|
||||
return (
|
||||
<AptabaseProvider appKey="<YOUR_APP_KEY>">
|
||||
<Component {...pageProps} />
|
||||
</AptabaseProvider>
|
||||
);
|
||||
}
|
||||
```
|
||||
|
||||
</details>
|
||||
|
||||
<details>
|
||||
<summary>Setup for Remix</summary>
|
||||
|
||||
Add `AptabaseProvider` to your `entry.client.tsx` file:
|
||||
|
||||
```tsx
|
||||
import { AptabaseProvider } from '@aptabase/react';
|
||||
import { RemixBrowser } from '@remix-run/react';
|
||||
import { startTransition, StrictMode } from 'react';
|
||||
import { hydrateRoot } from 'react-dom/client';
|
||||
|
||||
startTransition(() => {
|
||||
hydrateRoot(
|
||||
document,
|
||||
<StrictMode>
|
||||
<AptabaseProvider appKey="<YOUR_APP_KEY>">
|
||||
<RemixBrowser />
|
||||
</AptabaseProvider>
|
||||
</StrictMode>,
|
||||
);
|
||||
});
|
||||
```
|
||||
|
||||
</details>
|
||||
|
||||
<details>
|
||||
<summary>Setup for Create React App or Vite</summary>
|
||||
|
||||
Add `AptabaseProvider` to your root component:
|
||||
|
||||
```tsx
|
||||
import { AptabaseProvider } from '@aptabase/react';
|
||||
|
||||
ReactDOM.createRoot(root).render(
|
||||
<React.StrictMode>
|
||||
<AptabaseProvider appKey="<YOUR_APP_KEY>">
|
||||
<YourApp />
|
||||
</AptabaseProvider>
|
||||
</React.StrictMode>,
|
||||
);
|
||||
```
|
||||
|
||||
</details>
|
||||
|
||||
## Advanced setup
|
||||
|
||||
The `AptabaseProvider` also supports an optional second parameter, which is an object with the `appVersion` property.
|
||||
|
||||
It's up to you to decide how to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time. Alternatively you can also pass it in manually.
|
||||
|
||||
It's up to you to decide what to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time.
|
||||
|
||||
## Tracking Events with Aptabase
|
||||
|
||||
After setting up the `AptabaseProvider`, you can then start tracking events using the `useAptabase` hook.
|
||||
|
||||
Here's an example of a simple counter component that tracks the `increment` and `decrement` events:
|
||||
|
||||
```js
|
||||
'use client';
|
||||
|
||||
import { useState } from 'react';
|
||||
import { useAptabase } from '@aptabase/react';
|
||||
|
||||
export function Counter() {
|
||||
const { trackEvent } = useAptabase();
|
||||
const [count, setCount] = useState(0);
|
||||
|
||||
function increment() {
|
||||
setCount((c) => c + 1);
|
||||
trackEvent('increment', { count });
|
||||
}
|
||||
|
||||
function decrement() {
|
||||
setCount((c) => c - 1);
|
||||
trackEvent('decrement', { count });
|
||||
}
|
||||
|
||||
return (
|
||||
<div>
|
||||
<p>Count: {count}</p>
|
||||
<button onClick={increment}>Increment</button>
|
||||
<button onClick={decrement}>Decrement</button>
|
||||
</div>
|
||||
);
|
||||
}
|
||||
```
|
||||
|
||||
A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to await the `trackEvent` function, it'll run in the background.
|
||||
4. Only strings and numeric values are allowed on custom properties
|
|
@ -1,42 +0,0 @@
|
|||
{
|
||||
"name": "@aptabase/react",
|
||||
"version": "0.3.5",
|
||||
"type": "module",
|
||||
"description": "React SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"main": "./dist/index.cjs",
|
||||
"module": "./dist/index.js",
|
||||
"types": "./dist/index.d.ts",
|
||||
"exports": {
|
||||
".": {
|
||||
"require": "./dist/index.cjs",
|
||||
"import": "./dist/index.js",
|
||||
"types": "./dist/index.d.ts"
|
||||
}
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/aptabase/aptabase-js.git",
|
||||
"directory": "packages/react"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/aptabase/aptabase-js/issues"
|
||||
},
|
||||
"homepage": "https://github.com/aptabase/aptabase-js",
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"build": "tsup",
|
||||
"prepublishOnly": "npm run build"
|
||||
},
|
||||
"publishConfig": {
|
||||
"access": "public"
|
||||
},
|
||||
"files": [
|
||||
"README.md",
|
||||
"LICENSE",
|
||||
"dist",
|
||||
"package.json"
|
||||
],
|
||||
"peerDependencies": {
|
||||
"react": "^18.0.0"
|
||||
}
|
||||
}
|
|
@ -1,104 +0,0 @@
|
|||
'use client';
|
||||
|
||||
import { createContext, useContext, useEffect, useState } from 'react';
|
||||
import { getApiUrl, inMemorySessionId, sendEvent, validateAppKey, type AptabaseOptions } from '../../shared';
|
||||
|
||||
// Session expires after 1 hour of inactivity
|
||||
const SESSION_TIMEOUT = 1 * 60 * 60;
|
||||
const sdkVersion = `aptabase-react@${process.env.PKG_VERSION}`;
|
||||
|
||||
let _appKey = '';
|
||||
let _apiUrl: string | undefined;
|
||||
let _options: AptabaseOptions | undefined;
|
||||
let _eventsBeforeInit: { [key: string]: Record<string, string | number | boolean> | undefined } = {};
|
||||
|
||||
type ContextProps = {
|
||||
appKey?: string;
|
||||
options?: AptabaseOptions;
|
||||
isInitialized: boolean;
|
||||
};
|
||||
|
||||
function init(appKey: string, options?: AptabaseOptions) {
|
||||
if (!validateAppKey(appKey)) return;
|
||||
|
||||
_apiUrl = options?.apiUrl ?? getApiUrl(appKey, options);
|
||||
_appKey = appKey;
|
||||
_options = options;
|
||||
|
||||
Object.keys(_eventsBeforeInit).forEach((eventName) => {
|
||||
trackEvent(eventName, _eventsBeforeInit[eventName]);
|
||||
});
|
||||
_eventsBeforeInit = {};
|
||||
}
|
||||
|
||||
async function trackEvent(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
if (!_apiUrl) return;
|
||||
|
||||
const sessionId = inMemorySessionId(SESSION_TIMEOUT);
|
||||
|
||||
await sendEvent({
|
||||
apiUrl: _apiUrl,
|
||||
sessionId,
|
||||
appKey: _appKey,
|
||||
isDebug: _options?.isDebug,
|
||||
appVersion: _options?.appVersion,
|
||||
sdkVersion,
|
||||
eventName,
|
||||
props,
|
||||
});
|
||||
}
|
||||
|
||||
function trackEventBeforeInit(eventName: string, props?: Record<string, string | number | boolean>): Promise<void> {
|
||||
_eventsBeforeInit[eventName] = props;
|
||||
return Promise.resolve();
|
||||
}
|
||||
|
||||
const AptabaseContext = createContext<ContextProps>({ isInitialized: false });
|
||||
|
||||
type Props = {
|
||||
appKey: string;
|
||||
options?: AptabaseOptions;
|
||||
children: React.ReactNode;
|
||||
};
|
||||
|
||||
export { type AptabaseOptions };
|
||||
|
||||
export type AptabaseClient = {
|
||||
trackEvent: typeof trackEvent;
|
||||
};
|
||||
|
||||
export function AptabaseProvider({ appKey, options, children }: Props) {
|
||||
const [isInitialized, setIsInitialized] = useState(false);
|
||||
|
||||
useEffect(() => {
|
||||
init(appKey, options);
|
||||
setIsInitialized(true);
|
||||
}, [appKey, options]);
|
||||
|
||||
return <AptabaseContext.Provider value={{ appKey, options, isInitialized }}>{children}</AptabaseContext.Provider>;
|
||||
}
|
||||
|
||||
export function useAptabase(): AptabaseClient {
|
||||
const ctx = useContext(AptabaseContext);
|
||||
|
||||
if (!ctx.appKey) {
|
||||
return {
|
||||
trackEvent: () => {
|
||||
console.warn(
|
||||
`Aptabase: useAptabase must be used within AptabaseProvider. Did you forget to wrap your app in <AptabaseProvider>?`,
|
||||
);
|
||||
return Promise.resolve();
|
||||
},
|
||||
};
|
||||
}
|
||||
|
||||
if (!ctx.isInitialized) {
|
||||
return {
|
||||
trackEvent: trackEventBeforeInit,
|
||||
};
|
||||
}
|
||||
|
||||
return {
|
||||
trackEvent,
|
||||
};
|
||||
}
|
|
@ -1,15 +0,0 @@
|
|||
{
|
||||
"compilerOptions": {
|
||||
"target": "es6",
|
||||
"strict": true,
|
||||
"moduleResolution": "node",
|
||||
"esModuleInterop": true,
|
||||
"jsx": "react-jsx",
|
||||
"baseUrl": ".",
|
||||
"paths": {
|
||||
"types": ["@types"]
|
||||
},
|
||||
"declaration": true,
|
||||
"declarationDir": "./dist"
|
||||
}
|
||||
}
|
|
@ -1,15 +0,0 @@
|
|||
import { defineConfig } from 'tsup';
|
||||
const { version } = require('./package.json');
|
||||
|
||||
export default defineConfig({
|
||||
entry: ['src/index.tsx'],
|
||||
format: ['cjs', 'esm'],
|
||||
dts: true,
|
||||
splitting: false,
|
||||
minify: true,
|
||||
sourcemap: true,
|
||||
clean: true,
|
||||
env: {
|
||||
PKG_VERSION: version,
|
||||
},
|
||||
});
|
|
@ -1,16 +1,16 @@
|
|||
let defaultLocale: string | undefined;
|
||||
let defaultIsDebug: boolean | undefined;
|
||||
const isInBrowser = typeof window !== 'undefined' && typeof window.fetch !== 'undefined';
|
||||
const isInBrowserExtension = typeof chrome !== 'undefined' && !!chrome.runtime?.id;
|
||||
const isInBrowser = true;
|
||||
|
||||
let _sessionId = newSessionId();
|
||||
let _lastTouched = new Date();
|
||||
|
||||
const _hosts: { [region: string]: string } = {
|
||||
US: 'https://us.aptabase.com',
|
||||
EU: 'https://eu.aptabase.com',
|
||||
DEV: 'https://localhost:3000',
|
||||
SH: '',
|
||||
US: 'https://us.aptabase.com', // Operated by Aptabase in North America
|
||||
EU: 'https://eu.aptabase.com', // Operated by Aptabase in Europe
|
||||
SV: 'https://events.sudovanilla.org', // Owned and operated by SudoVanilla in North America
|
||||
DEV: 'https://localhost:3000', // Local Development
|
||||
SH: '', // Selfhost
|
||||
};
|
||||
|
||||
export type AptabaseOptions = {
|
||||
|
@ -80,7 +80,7 @@ export async function sendEvent(opts: {
|
|||
eventName: string;
|
||||
props?: Record<string, string | number | boolean>;
|
||||
}): Promise<void> {
|
||||
if (!isInBrowser && !isInBrowserExtension) {
|
||||
if (!isInBrowser) {
|
||||
console.warn(`Aptabase: trackEvent requires a browser environment. Event "${opts.eventName}" will be discarded.`);
|
||||
return;
|
||||
}
|
||||
|
|
|
@ -1,3 +1,28 @@
|
|||
# Zalvena
|
||||
## 0.5.0
|
||||
- Removed examples
|
||||
- Removed Eslintrc file
|
||||
- Removed package lock file
|
||||
- Removed Engines from `packages.json`
|
||||
- Removed `/.changeset/`
|
||||
- Removed `/.github/`
|
||||
- Removed `/.vscode/`
|
||||
- Removed other packages: `["angular", "react", "browser"]`
|
||||
- Removed `isInBrowserExtension` from `shared.ts`
|
||||
- Removed `isInBrowserExtension` from `shared.ts`
|
||||
- Updated license from MIT to MIT-NON-AI
|
||||
- Updated the package `@types/node` from `v20.5.7` to `v22.13.4`
|
||||
- Updated the package `prettier` from `v3.0.3` to `v3.5.1`
|
||||
- Updated the package `tsup` from `v7.2.0` to `v8.3.6`
|
||||
- Updated the package `turbo` from `v1.10.13` to `v2.4.2`
|
||||
- Added SudoVanilla's Aptabase instance to `regions` list as `SV`
|
||||
- Using Bun over NPM
|
||||
|
||||
You can view other changes by viewing the commit history.
|
||||
|
||||
___
|
||||
# Officially from Aptabase
|
||||
|
||||
## 0.4.3
|
||||
|
||||
- Move browser locale and is debug reads from import to first event to better support SSR
|
||||
|
|
|
@ -1,21 +1,15 @@
|
|||
MIT License
|
||||
|
||||
Copyright (c) 2023 Sumbit Labs Ltd.
|
||||
Copyright 2025 SudoVanilla
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the “Software”), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
In addition, the following restrictions apply:
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
||||
1. The Software and any modifications made to it may not be used for the purpose of training or improving machine learning algorithms, including but not limited to artificial intelligence, natural language processing, or data mining. This condition applies to any derivatives, modifications, or updates based on the Software code. Any usage of the Software in an AI-training dataset is considered a breach of this License.
|
||||
|
||||
2. The Software may not be included in any dataset used for training or improving machine learning algorithms, including but not limited to artificial intelligence, natural language processing, or data mining.
|
||||
|
||||
3. Any person or organization found to be in violation of these restrictions will be subject to legal action and may be held liable for any damages resulting from such use.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
@ -1,52 +0,0 @@
|
|||
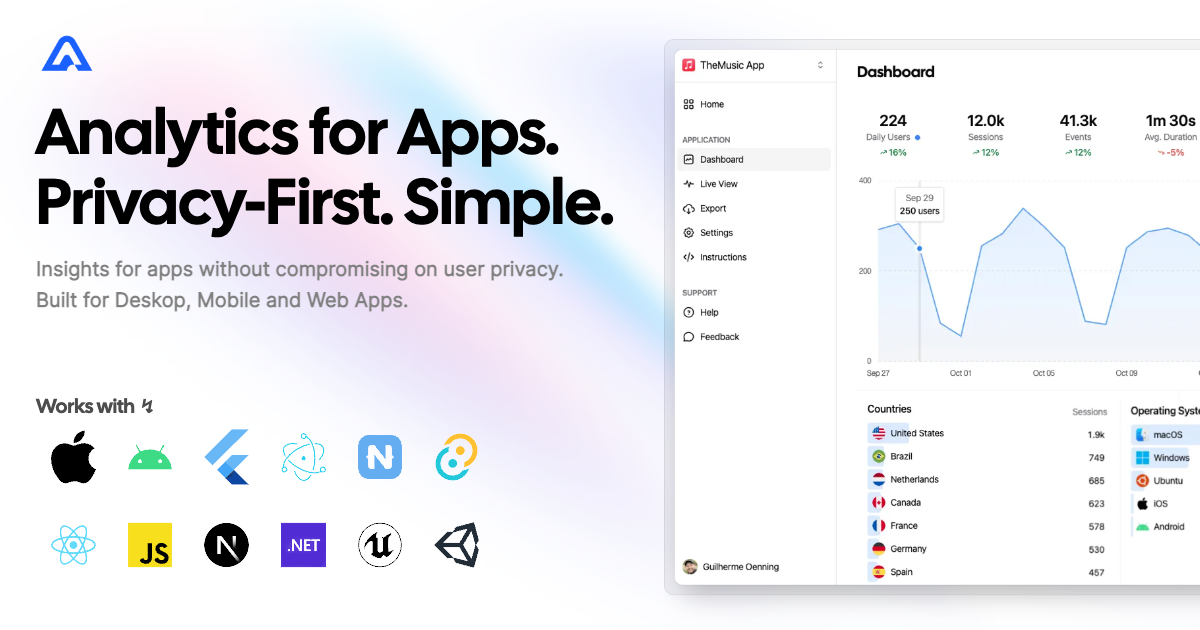
|
||||
|
||||
# Aptabase SDK for Web Apps
|
||||
|
||||
A tiny SDK (1 kB) to instrument your web app with Aptabase, an Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps.
|
||||
|
||||
Building a React app? Use the `@aptabase/react` package instead.
|
||||
|
||||
> 👉 **IMPORTANT**
|
||||
>
|
||||
> This SDK is for **Web Applications**, not websites. There's a subtle, but important difference. A web app is often a lot more interactive and does not cause a full page reload when the user interacts with it. It's often called a **Single-Page Application**. A website, on the other hand, is a lot more content-focused like marketing sites, landing pages, blogs, etc. While you can certainly use Aptabase to track events on websites, please be aware that each page reload will be considered a new session.
|
||||
|
||||
## Install
|
||||
|
||||
Install the SDK using npm or your preferred JavaScript package manager
|
||||
|
||||
```bash
|
||||
npm add @aptabase/web
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
First you need to get your `App Key` from Aptabase, you can find it in the `Instructions` menu on the left side menu.
|
||||
|
||||
Initialize the SDK using your `App Key`:
|
||||
|
||||
```js
|
||||
import { init } from '@aptabase/web';
|
||||
|
||||
init('<YOUR_APP_KEY>'); // 👈 this is where you enter your App Key
|
||||
```
|
||||
|
||||
The init function also supports an optional second parameter, which is an object with the `appVersion` property.
|
||||
|
||||
It's up to you to decide how to get the version of your app, but it's generally recommended to use your bundler (like Webpack, Vite, Rollup, etc.) to inject the values at build time. Alternatively you can also pass it in manually.
|
||||
|
||||
Afterwards you can start tracking events with `trackEvent`:
|
||||
|
||||
```js
|
||||
import { trackEvent } from '@aptabase/web';
|
||||
|
||||
trackEvent('connect_click'); // An event with no properties
|
||||
trackEvent('play_music', { name: 'Here comes the sun' }); // An event with a custom property
|
||||
```
|
||||
|
||||
A few important notes:
|
||||
|
||||
1. The SDK will automatically enhance the event with some useful information, like the OS and other properties.
|
||||
2. You're in control of what gets sent to Aptabase. This SDK does not automatically track any events, you need to call `trackEvent` manually.
|
||||
- Because of this, it's generally recommended to at least track an event at startup
|
||||
3. You do not need to await the `trackEvent` function, it'll run in the background.
|
||||
4. Only strings and numeric values are allowed on custom properties
|
|
@ -1,8 +1,8 @@
|
|||
{
|
||||
"name": "@aptabase/web",
|
||||
"version": "0.4.3",
|
||||
"name": "@sudovanilla/zalvena",
|
||||
"version": "1.0.0",
|
||||
"type": "module",
|
||||
"description": "JavaScript SDK for Aptabase: Open Source, Privacy-First and Simple Analytics for Mobile, Desktop and Web Apps",
|
||||
"description": "JavaScript SDK for Aptabase",
|
||||
"main": "./dist/index.cjs",
|
||||
"module": "./dist/index.js",
|
||||
"types": "./dist/index.d.ts",
|
||||
|
@ -15,20 +15,19 @@
|
|||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/aptabase/aptabase-js.git",
|
||||
"url": "git+https://ark.sudovanilla.org/korbs/zalvena",
|
||||
"directory": "packages/web"
|
||||
},
|
||||
"bugs": {
|
||||
"url": "https://github.com/aptabase/aptabase-js/issues"
|
||||
"url": "https://ark.sudovanilla.org/korbs/zalvena/issues"
|
||||
},
|
||||
"homepage": "https://github.com/aptabase/aptabase-js",
|
||||
"homepage": "https://ark.sudovanilla.org/korbs/zalvena",
|
||||
"license": "MIT",
|
||||
"scripts": {
|
||||
"build": "tsup",
|
||||
"prepublishOnly": "npm run build"
|
||||
"prepublishOnly": "bun run build"
|
||||
},
|
||||
"files": [
|
||||
"README.md",
|
||||
"LICENSE",
|
||||
"dist",
|
||||
"package.json"
|
||||
|
|
|
@ -1,6 +1,6 @@
|
|||
{
|
||||
"compilerOptions": {
|
||||
"target": "es2020",
|
||||
"target": "es2022",
|
||||
"strict": true,
|
||||
"moduleResolution": "node",
|
||||
"esModuleInterop": true,
|
||||
|
|
Loading…
Add table
Add a link
Reference in a new issue