diff --git a/core/server/services/members/importer/email-template.js b/core/server/services/members/importer/email-template.js
deleted file mode 100644
index 2c88b3cfce..0000000000
--- a/core/server/services/members/importer/email-template.js
+++ /dev/null
@@ -1,178 +0,0 @@
-const iff = (cond, yes, no) => (cond ? yes : no);
-module.exports = ({result, siteUrl, membersUrl, emailRecipient}) => `
-
-
-
-
-
- |
-
-
-
-
-
-
-
-
-
-
-
-
- 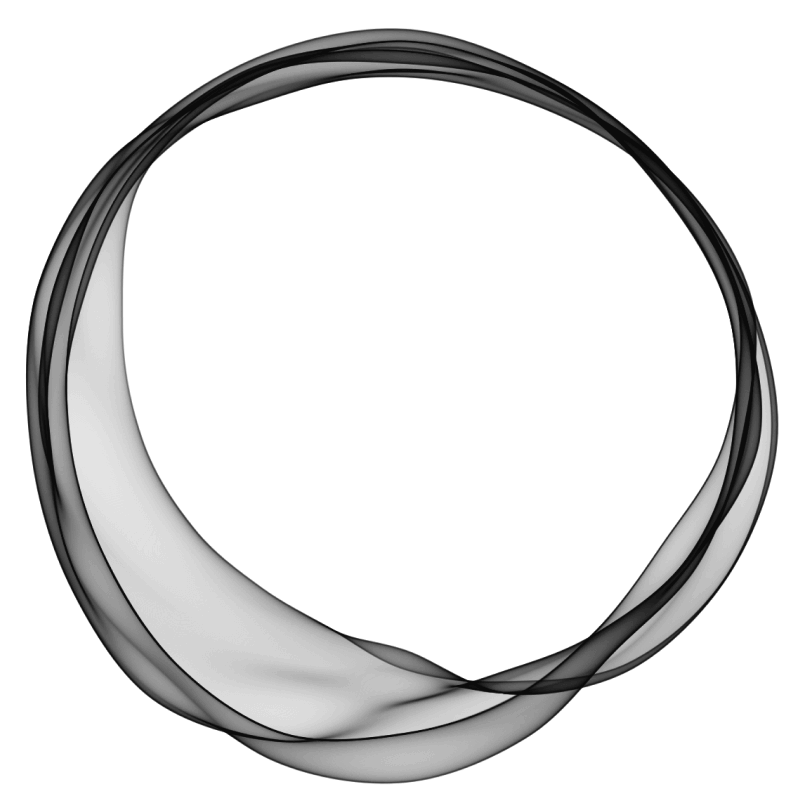 |
-
-
-
- ${iff(result.imported > 0, `Your member import is complete`, `Your member import was unsuccessful`)}
- |
-
- ${iff(result.imported === 0 && result.errors.length === 0, `
-
-
- No members were added.
- |
-
- `, ``)}
- ${iff(result.imported > 0, `
-
-
- A total of ${result.imported} ${iff(result.imported === 1, 'person', 'people')} were successfully added or updated in your list of members, and now have access to your site.
- |
- `, ``)}
- ${iff(result.errors.length > 0, `
-
-
-
- ${iff(result.imported === 0, `No members were added.`, `${result.errors.length} ${iff(result.errors.length === 1, `member was`, `members were`)} skipped due to errors.`)} There's a validated CSV file attached to this email with the list of errors so that you can fix them and re-upload the CSV to complete the import.
- |
- `, '')}
-
-
- ${iff(result.imported > 0, `View members`, `Try again`)}
- |
-
-
- |
-
-
-
-
- |
-
-
-
-
-
-
-
-
- |
- |
-
-
-
-
-`;
-
diff --git a/core/server/services/members/importer/importer.js b/core/server/services/members/importer/importer.js
deleted file mode 100644
index c905f3aebe..0000000000
--- a/core/server/services/members/importer/importer.js
+++ /dev/null
@@ -1,316 +0,0 @@
-const moment = require('moment-timezone');
-const path = require('path');
-const fs = require('fs-extra');
-const membersCSV = require('@tryghost/members-csv');
-const errors = require('@tryghost/errors');
-const tpl = require('@tryghost/tpl');
-
-const emailTemplate = require('./email-template');
-
-const messages = {
- filenameCollision: 'Filename already exists, please try again.',
- jobAlreadyComplete: 'Job is already complete.'
-};
-
-module.exports = class MembersCSVImporter {
- /**
- * @param {Object} options
- * @param {string} options.storagePath - The path to store CSV's in before importing
- * @param {Function} options.getTimezone - function returning currently configured timezone
- * @param {() => Object} options.getMembersApi
- * @param {Function} options.sendEmail - function sending an email
- * @param {(string) => boolean} options.isSet - Method checking if specific feature is enabled
- * @param {({name, at, job, data, offloaded}) => void} options.addJob - Method registering an async job
- * @param {Object} options.knex - An instance of the Ghost Database connection
- * @param {Function} options.urlFor - function generating urls
- */
- constructor({storagePath, getTimezone, getMembersApi, sendEmail, isSet, addJob, knex, urlFor}) {
- this._storagePath = storagePath;
- this._getTimezone = getTimezone;
- this._getMembersApi = getMembersApi;
- this._sendEmail = sendEmail;
- this._isSet = isSet;
- this._addJob = addJob;
- this._knex = knex;
- this._urlFor = urlFor;
- }
-
- /**
- * @typedef {string} JobID
- */
-
- /**
- * @typedef {Object} Job
- * @prop {string} filename
- * @prop {JobID} id
- * @prop {string} status
- */
-
- /**
- * Get the Job for a jobCode
- * @param {JobID} jobId
- * @returns {Promise